java高德通过经纬度获取地址
时间: 2024-09-11 16:18:36 浏览: 58
在Java中,你可以利用高德地图API来通过经纬度获取地址信息。首先,你需要在官网(https://lbs.amap.com/api)注册并获取到高德地图的Key。然后,可以使用`AMapGeocoder`类进行逆地理编码操作。
以下是一个简单的示例:
```java
import com.amap.api.location.AMapLocation;
import com.amap.api.location.AMapLocationClient;
import com.amap.api.location.AMapLocationClientOption;
import com.amap.api.services.geocoding.GeocodeSearch;
import com.amap.api.services.geocoding.GeocodeSearchResponse;
import com.amap.api.services.geocoding.RegeocodeQuery;
public class GeocodingExample {
private static final String YOUR_AMAP_KEY = "your_amap_key_here";
public static void main(String[] args) {
// 初始化定位客户端
AMapLocationClient locationClient = new AMapLocationClient(context);
AMapLocationClientOption option = new AMapLocationClientOption();
// 设置定位超时时间
option.setLocateTimeout(1000 * 60); // 单位:毫秒,60秒
// 开启高精度定位
option.setIsNeedAddress(true);
option.setNeedLocationDescribe(true);
locationClient.setLocationOption(option);
RegeocodeQuery query = new RegeocodeQuery(); // 创建逆地理编码查询对象
query.setAddress("经度,纬度"); // 给定经纬度
query.setCitycode("城市码"); // 如果需要指定城市,默认值为当前城市
GeocodeSearch geocodeSearch = new GeocodeSearch(context, YOUR_AMAP_KEY);
geocodeSearch.setOnGetRegeocodeResultListener(new GeocodeSearch.OnGetRegeocodeResultListener() {
@Override
public void onGetRegeocodeResult(GeocodeSearchResponse response) {
if (response != null && response.regeocode != null) {
AMapGeoPoint point = response.regeocode.result.getGeometry().getPoint();
String address = response.regeocode.addressComponent.getStreetName();
System.out.println("地理位置:" + point + ", 地址:" + address);
} else {
System.out.println("未找到结果");
}
}
@Override
public void onGetError(int errorType) {
System.out.println("获取地点失败,错误码:" + errorType);
}
});
// 发起逆地理编码请求
geocodeSearch.search(query);
}
}
```
在这个例子中,你需要替换`YOUR_AMAP_KEY`为你实际的API Key,并根据需求调整经纬度、城市码等参数。运行此代码后,它会返回给定经纬度对应的详细地址。
相关推荐
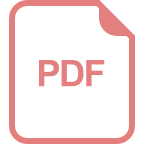
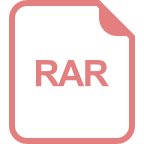
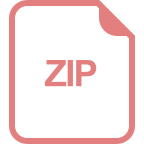


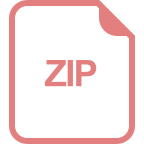
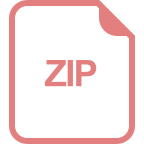
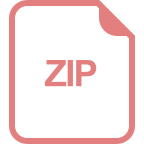










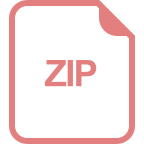