python员工考勤管理系统
时间: 2023-10-21 09:06:55 浏览: 81
对于Python员工考勤管理系统的开发,可以采用以下步骤:
1. 定义员工类:创建一个Employee类,包含员工的基本信息,如姓名、工号等。
```python
class Employee:
def __init__(self, name, emp_id):
self.name = name
self.emp_id = emp_id
```
2. 定义考勤记录类:创建一个Attendance类,用于记录员工的考勤信息,如日期、上班时间、下班时间等。
```python
class Attendance:
def __init__(self, date, start_time, end_time):
self.date = date
self.start_time = start_time
self.end_time = end_time
```
3. 实现考勤管理功能:创建一个AttendanceManagement类,用于管理员工的考勤记录。
```python
class AttendanceManagement:
def __init__(self):
self.attendance_records = []
def add_attendance_record(self, attendance):
self.attendance_records.append(attendance)
def get_attendance_records(self):
return self.attendance_records
```
4. 使用系统:使用上述定义的类进行员工考勤管理系统的操作,例如添加考勤记录、查询考勤记录等。
```python
# 创建员工对象
emp1 = Employee("John", "001")
# 创建考勤记录对象
attendance1 = Attendance("2022-01-01", "09:00", "18:00")
# 创建考勤管理对象
attendance_management = AttendanceManagement()
# 添加考勤记录到考勤管理系统
attendance_management.add_attendance_record(attendance1)
# 查询考勤记录
records = attendance_management.get_attendance_records()
for record in records:
print(f"Date: {record.date}, Start Time: {record.start_time}, End Time: {record.end_time}")
```
相关推荐
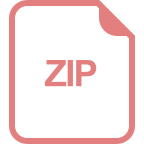
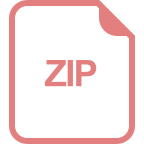
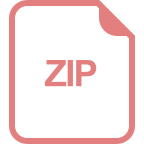

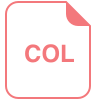












