c# string 截取第一个空格 分成2个string
时间: 2024-11-03 18:22:47 浏览: 19
在 C# 中,你可以使用 `Substring` 方法结合 `IndexOf` 方法来截取字符串并找到第一个空格的位置,然后创建一个新的子字符串。以下是一个示例:
```csharp
string inputString = "Example String";
int spaceIndex = inputString.IndexOf(' ');
if (spaceIndex != -1) { // 检查是否有空格
string firstPart = inputString.Substring(0, spaceIndex); // 截取到第一个空格前的部分
string secondPart = inputString.Substring(spaceIndex + 1); // 截取从第一个空格后的部分
Console.WriteLine($"First part: {firstPart}");
Console.WriteLine($"Second part: {secondPart}");
} else {
Console.WriteLine("No spaces found in the string.");
}
```
这段代码首先查找字符串中的第一个空格(' '),如果找到了,就分别获取到空格前的子串(`firstPart`)和空格后的子串(`secondPart`)。如果没有找到空格,则输出提示。
相关问题
c# string按空格截取字符串
在 C# 中,如果你想按照空格将字符串分割成多个部分,你可以使用 `Split` 方法。这个方法会返回一个字符串数组,其中包含原始字符串中所有以空格分隔的部分。下面是一个简单的示例:
```csharp
string originalString = "Hello World, This is a test.";
string[] parts = originalString.Split(' '); // 使用 ' ' (空格字符) 作为分隔符
foreach (string part in parts)
{
Console.WriteLine(part);
}
```
在这个例子中,`parts` 数组将会是 `["Hello", "World,", "This", "is", "a", "test."]`。
如果你想要从字符串的开头开始截取,直到遇到第一个空格,可以使用 `Substring` 和 `IndexOf` 方法结合:
```csharp
int index = originalString.IndexOf(' ');
if (index != -1)
{
string firstPart = originalString.Substring(0, index);
Console.WriteLine(firstPart); // 输出 "Hello"
}
```
c#中一个string类型数据如何获取空格前的数据
在C#中,要获取一个字符串中空格前的数据,可以使用`String.IndexOf`方法找到第一个空格的位置,然后利用`String.Substring`方法截取空格前的子字符串。以下是具体的实现步骤:
1. 使用`IndexOf`方法确定第一个空格字符的索引位置。
2. 检查索引是否不等于-1(表示字符串中确实存在空格)。
3. 使用`Substring`方法从字符串的开始位置截取到空格的位置(不包含空格字符本身)。
示例代码如下:
```csharp
string originalString = "Example string data";
int spaceIndex = originalString.IndexOf(' '); // 获取第一个空格的索引
if (spaceIndex != -1)
{
string substringBeforeSpace = originalString.Substring(0, spaceIndex); // 截取空格前的字符串
Console.WriteLine(substringBeforeSpace); // 输出结果
}
else
{
Console.WriteLine("String does not contain a space.");
}
```
阅读全文
相关推荐
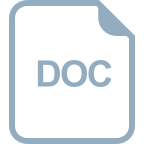
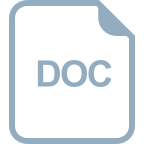
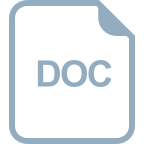





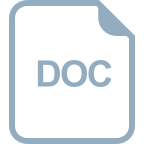
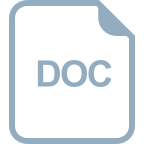
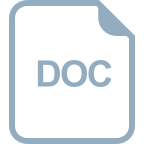
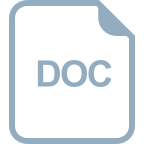



