python多线程爬虫实例
时间: 2024-05-20 08:09:18 浏览: 20
Python多线程爬虫是一个常见的应用场景,可以有效地提高爬取数据的效率。下面给您介绍一个简单的Python多线程爬虫实例:
```python
import requests
from threading import Thread
def get_content(url):
r = requests.get(url)
print(r.content)
urls = ['http://www.baidu.com', 'http://www.google.com', 'http://www.github.com']
for url in urls:
t = Thread(target=get_content, args=(url,))
t.start()
```
以上代码中,我们定义了一个 `get_content` 函数,用来向指定的url发送请求并打印响应内容。然后定义了一个url列表,遍历这个列表,将每个url作为参数传递给 `get_content` 函数,然后使用多线程的方式启动该函数。
这里使用 `Thread` 类创建线程,并使用 `start` 方法启动线程,其中 `target` 参数指定要执行的函数,`args` 参数是该函数的参数。这样,我们就可以同时向多个网站发送请求并获取响应内容。
相关问题
python多线程爬虫多个url实例解析
可以使用Python的多线程模块`threading`来实现多线程爬虫。以下是一个示例代码,可以同时爬取多个URL,并将每个URL的实例解析结果保存到一个列表中:
```python
import requests
import threading
from bs4 import BeautifulSoup
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
def get_data(url, results):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析实例,将结果存入结果列表
results.append(soup.find_all('div', {'class': 'example'}))
def main():
results = []
threads = []
# 创建线程
for url in urls:
t = threading.Thread(target=get_data, args=(url, results))
threads.append(t)
t.start()
# 等待所有线程完成
for t in threads:
t.join()
# 输出结果
print(results)
if __name__ == '__main__':
main()
```
在该示例中,我们首先定义了一个URL列表,然后定义了一个`get_data`函数,该函数以URL和结果列表作为参数。在函数中,我们使用`requests`库获取URL的HTML内容,并使用`BeautifulSoup`库解析实例。最后,将解析结果存入结果列表中。
在主函数中,我们创建多个线程,并将它们添加到线程列表中。然后,我们启动所有线程,并使用`join`方法等待它们完成。最后,我们输出结果列表。
注意,多线程爬虫可能会被目标网站视为恶意行为,因此在使用之前请确保已经了解目标网站的使用政策。
python多线程爬虫大作业-Python多线程爬虫简单示例
好的,下面是一个简单的Python多线程爬虫示例:
```python
import requests
import threading
from queue import Queue
# 定义一个全局变量用来存储爬取到的数据
data = []
# 定义一个线程锁,防止多个线程同时写入数据导致数据错乱
lock = threading.Lock()
# 定义一个队列,用来存储待爬取的URL
url_queue = Queue()
# 定义一个爬取线程类
class CrawlerThread(threading.Thread):
def __init__(self, url_queue):
super().__init__()
self.url_queue = url_queue
def run(self):
while True:
# 从队列中获取一个URL
url = self.url_queue.get()
try:
# 发送请求并解析响应数据
response = requests.get(url)
content = response.text
# 对响应数据进行处理(此处省略)
# ...
# 将处理后的数据存入全局变量
with lock:
data.append(processed_data)
# 标记该URL已被处理
self.url_queue.task_done()
except Exception as e:
print(f"Error occurred while crawling {url}: {e}")
# 如果发生错误,将该URL重新放回队列
self.url_queue.put(url)
self.url_queue.task_done()
# 定义一个入口函数
def main():
# 初始化待爬取的URL列表
urls = ["http://www.example.com/page{}".format(i) for i in range(1, 11)]
# 将URL列表添加到队列中
for url in urls:
url_queue.put(url)
# 创建多个爬取线程并启动
for i in range(5):
t = CrawlerThread(url_queue)
t.start()
# 阻塞主线程,直到所有URL都被处理完毕
url_queue.join()
# 输出爬取结果
print(data)
if __name__ == '__main__':
main()
```
以上示例中,我们定义了一个`CrawlerThread`类来表示爬取线程,定义了一个`url_queue`队列来存储待爬取的URL,定义了一个`data`列表来存储爬取到的数据,以及定义了一个`lock`线程锁来保证多个线程访问`data`时不会出现数据错乱的情况。
在`main`函数中,我们首先将待爬取的URL列表添加到`url_queue`队列中,然后创建多个`CrawlerThread`实例并启动它们。最后,我们使用`url_queue.join()`方法来阻塞主线程,直到所有的URL都被处理完毕。
在`CrawlerThread`类的`run`方法中,我们使用`self.url_queue.get()`方法从`url_queue`队列中获取一个URL,然后发送请求并解析响应数据。如果处理过程中出现错误,我们将该URL重新放回队列中,以便后续线程重新处理。如果处理成功,我们将处理后的数据存入`data`列表中,并使用`self.url_queue.task_done()`方法标记该URL已被处理完毕。
需要注意的是,在多线程爬虫中,如果爬取的网站对IP访问频率有限制,可能会导致IP被封禁的情况。因此,在实际应用中,我们需要合理设置线程数量和请求频率,以避免被封禁的情况发生。
相关推荐
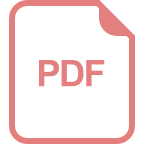
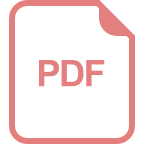












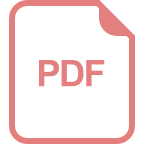