js map循环终止
时间: 2023-11-18 20:51:11 浏览: 36
在JavaScript中,使用map循环时,默认情况下是无法手动终止循环的。map循环将会对数组中的每个元素进行操作,并返回一个新的数组。如果你希望在特定条件下终止map循环,可以使用forEach循环或普通的for循环来代替。在forEach循环或普通for循环中,可以使用return语句来提前终止循环。
例如,在你提供的代码中,如果你希望在某个条件满足时终止循环,可以使用forEach循环或普通for循环:
使用forEach循环:
```
this.state.tab.forEach((res) => {
if (res.name.includes(this.state.value)) {
console.log(123);
} else {
console.log(1234);
}
// 在特定条件下终止循环
if (someCondition) {
return;
}
});
```
使用普通for循环:
```
for (let i = 0; i < this.state.tab.length; i++) {
const res = this.state.tab[i];
if (res.name.includes(this.state.value)) {
console.log(123);
} else {
console.log(1234);
}
// 在特定条件下终止循环
if (someCondition) {
break;
}
}
```
相关问题
js终止map循环
可以使用`break`关键字来终止`map`循环。但是`map`方法本身不支持`break`关键字,所以你需要使用其他的方法来模拟`map`循环并实现`break`的效果。例如,你可以使用`for...of`循环来遍历数组,并在需要的时候使用`break`关键字终止循环。以下是一个示例代码:
```javascript
const arr = [1, 2, 3, 4, 5];
for (const item of arr) {
if (item === 3) {
break;
}
console.log(item);
}
```
在上面的代码中,我们使用`for...of`循环遍历数组`arr`,并在遇到值为`3`的元素时使用`break`关键字终止循环。
js 如何终止map循环
在 JavaScript 中,可以使用 `Array.prototype.some()` 方法来终止 `Array.prototype.map()` 方法的循环。当 `Array.prototype.some()` 方法返回 `true` 时,`Array.prototype.map()` 方法的循环会被终止。例如:
```
const arr = [1, 2, 3, 4, 5];
const result = arr.map(item => {
if (item === 3) {
return item;
}
return item * 2;
}).some(item => item === 3);
console.log(result); // true
```
在上面的例子中,当 `item` 等于 3 时,`map()` 方法返回该值并终止循环,然后 `some()` 方法返回 `true`,从而终止 `map()` 方法的循环。
相关推荐
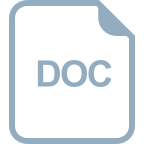
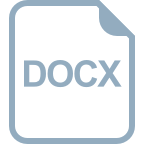
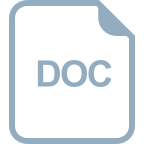







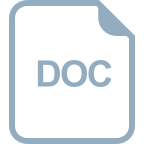
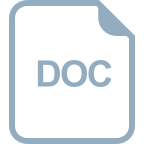
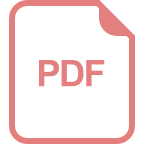
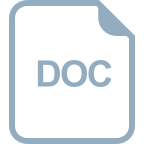
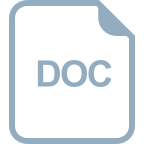