问题 5. 参照日期类,自己设计一个时间类,能初始化,可以输出,进行时间相减等操作 说明:时间的表示可以自行拟定,一般是”时分秒”形式,可以增加毫秒,采用 12或 24 小时‘ 部可以。用c++实现
时间: 2024-12-06 22:23:45 浏览: 20
在C++中,我们可以创建一个名为`Time`的时间类,用于表示小时、分钟、秒和毫秒。这里是一个简单的实现,我们以24小时制为基础,并支持时间相减:
```cpp
#include <iostream>
#include <chrono>
class Time {
private:
int hours;
int minutes;
int seconds;
int milliseconds;
public:
// 构造函数
Time(int h = 0, int m = 0, int s = 0, int ms = 0) : hours(h), minutes(m), seconds(s), milliseconds(ms) {}
// 输出时间
void display() const {
std::cout << hours << ":" << minutes << ":" << seconds << "." << milliseconds << "h" << std::endl;
}
// 添加时间(假设输入的是另一个Time对象)
Time add(const Time& other) const {
int total_hours = hours + other.hours;
int total_minutes = minutes + other.minutes;
int total_seconds = seconds + other.seconds;
int total_ms = milliseconds + other(milliseconds);
if (total_ms >= 1000) {
total_hours += total_ms / 1000;
total_ms %= 1000;
}
if (total_hours >= 24) {
total_hours -= 24;
}
return Time(total_hours, total_minutes % 60, total_seconds % 60, total_ms);
}
// 时间相减
Time subtract(const Time& other) const {
int hours_diff = hours - other.hours;
int min_diff = minutes - other.minutes;
// 如果第一个时刻晚于第二个,调整负数差异并计算总秒数
if (hours_diff < 0 || (hours_diff == 0 && minutes_diff < 0)) {
hours_diff += 24;
min_diff += 60;
}
int total_seconds = (hours_diff * 60 + min_diff) * 60 + seconds - other.seconds;
int ms_diff = milliseconds - other(milliseconds);
return Time(hours_diff, min_diff % 60, total_seconds % 60, ms_diff);
}
private:
// 私有函数,防止直接修改毫秒值
int milliseconds(int new_ms) const {
return (milliseconds + new_ms) % 1000;
}
};
int main() {
Time t1(12, 30, 45, 500); // 12:30:45.500h
Time t2(9, 45, 0, 0); // 9:45:00h
t1.display();
t2.display();
Time combined = t1.add(t2);
combined.display();
Time difference = t1.subtract(t2);
difference.display();
return 0;
}
```
这个例子中,`Time`类包含四个私有成员变量(小时、分钟、秒和毫秒),以及构造函数、显示方法、加法和减法操作。注意时间相减可能涉及到跨天的情况,这里通过调整小时数来处理。
阅读全文
相关推荐
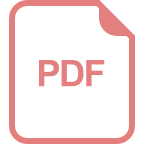
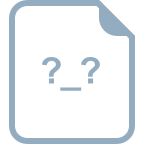















