编写一个vue商品列表,上方有一个输入框和搜索按钮,打开页面,鼠标默认放在搜索框中,搜索框中默认文字为“请输入关键字”,且搜索框中文本颜色为灰色
时间: 2024-10-22 16:10:22 浏览: 16
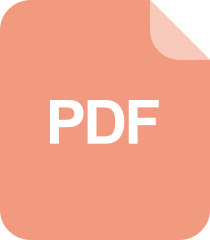
Vue.js页面中有多个input搜索框如何实现防抖操作
要编写一个Vue.js的商品列表组件,首先需要安装Vue CLI并创建一个新的项目。在这个项目中,我们将创建一个`ProductList.vue`文件来构建这个功能。以下是基本的组件结构以及相关的样式设置:
```html
<template>
<div class="product-list-container">
<input type="text" v-model="searchKeyword" @keyup.enter="searchProducts"
:placeholder="placeholderText" :class="{ greyText: !isSearching }"
ref="searchInput">
<button @click="searchProducts" :disabled="!searchKeyword">搜索</button>
<ul v-for="(product, index) in filteredProducts" :key="index">
<!-- 商品列表项 -->
<li>{{ product.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
searchKeyword: '',
products: [], // 假设这是从API获取的商品数组
placeholderText: '请输入关键字',
isSearching: false,
};
},
methods: {
searchProducts() {
if (this.searchKeyword.trim()) {
this.isSearching = true;
// 这里你可以发送请求到API搜索关键词匹配的产品
// 比如:this.$axios.get('/api/search', { params: { keyword: this.searchKeyword } });
// 在实际应用中替换为实际的查询逻辑,并更新filteredProducts数据
} else {
this.filteredProducts = this.products; // 如果没有关键词,则显示所有产品
}
},
},
computed: {
filteredProducts() {
return this.products.filter(product =>
product.name.includes(this.searchKeyword));
},
},
};
</script>
<style scoped>
.product-list-container {
display: flex;
align-items: center;
}
.greyText {
color: gray;
}
</style>
```
在这个例子中,我们使用了Vue的v-model指令绑定搜索框的内容,并监听keyup事件来触发搜索方法。当用户点击搜索按钮或按下回车键时,会检查输入是否为空,然后根据输入内容过滤商品列表。
注意这只是一个基础示例,实际应用中还需要处理异步数据获取、错误处理等情况。同时,样式部分使用了Vue的scoped属性,确保CSS仅应用于当前组件。如果你想要自定义更复杂的搜索效果,可以进一步调整CSS样式。
阅读全文
相关推荐
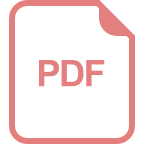
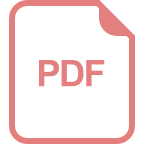
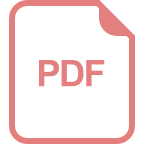












