javaweb网站计数器
时间: 2024-06-30 19:01:19 浏览: 175
Java Web网站计数器是一个常见的编程练习,用于演示如何使用会话(Session)或cookie来追踪用户访问次数。基本的实现步骤如下:
1. **使用HTTP会话(Session)**:
- 当用户访问网站时,服务器创建一个新的会话并存储一个计数值(例如默认为0)。
- 如果用户已经登录,检查会话中是否存在该计数值,存在则加1,不存在则初始化为1。
- 用户首次访问时,服务器设置一个名为"counter"的cookie,值为1。
- 每次用户访问,服务器读取cookie中的值加1,然后更新cookie。
- 但是,Cookie不持久存储,浏览器关闭后会丢失计数。
3. **数据库存储**:
- 更复杂的做法是将计数信息存储在数据库中,每次访问查询并更新计数。
4. **安全性**:
- 考虑到安全性和防止恶意刷新,可以设置一些限制条件,比如设定一段时间内的刷新间隔。
相关问题:
1. 如何处理多个用户的并发访问以保证计数准确性?
2. 在Java Web中,如果用户禁用了Cookie,计数器应该如何实现?
3. 使用HTTP会话存储计数数据时,如何避免会话过期导致的计数丢失?
相关问题
javaweb制作一个网站计数器。点击刷新,数值发生变化
创建一个简单的javaweb网站计数器,可以使用Servlet和JSP技术来实现。以下是基本步骤:
1. **设置项目结构**:在Eclipse或IntelliJ等IDE中,创建一个新的Web项目,并将计数器功能放在一个Servlet(例如CounterServlet.java)和一个相关的JSP文件(如counter.jsp)中。
2. **编写CounterServlet**:这个Servlet负责处理用户的请求和计数。每次用户点击刷新,需要增加计数器的值。你可以使用`HttpSession`来存储计数,因为它在会话期间有效,示例代码如下:
```java
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class CounterServlet extends HttpServlet {
private int count = 0;
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.getSession().setAttribute("counter", ++count);
RequestDispatcher rd = getServletContext().getRequestDispatcher("/counter.jsp");
rd.forward(request, response);
}
}
```
3. **设计counter.jsp**:这是一个动态生成的页面,从Servlet获取计数并显示。你可以使用EL表达式来访问session属性:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>计数器</title>
</head>
<body>
<h1>点击次数:${sessionScope.counter}</h1>
<a href="<c:url value='/CounterServlet'>刷新计数</c:url>">刷新</a>
</body>
</html>
```
4. **部署应用**:将Servlet和JSP文件打包到war文件中,然后部署到Tomcat、Jetty或其他Java Web服务器上。
5. **测试**:通过浏览器访问应用,点击“刷新”链接,你应该能看到计数器的值递增。
javaweb中使用文件存储方式实现网站计数器功能。
在Java Web中,可以使用文件存储方式来实现网站计数器的功能。具体实现步骤如下:
1. 在Web应用程序的根目录下创建一个名为“counter”的文件夹,用于存储计数器文件。
2. 在Servlet中使用以下代码来读取计数器文件的值:
```java
String filePath = application.getRealPath("/counter/count.txt"); // 获取计数器文件的路径
File file = new File(filePath); // 创建File对象
int count = 0; // 初始化计数器为0
if (file.exists()) { // 如果文件存在
BufferedReader br = new BufferedReader(new FileReader(file)); // 创建BufferedReader对象
count = Integer.parseInt(br.readLine()); // 读取文件中的计数器值
br.close(); // 关闭BufferedReader
}
```
3. 在Servlet中使用以下代码来更新计数器文件的值:
```java
String filePath = application.getRealPath("/counter/count.txt"); // 获取计数器文件的路径
File file = new File(filePath); // 创建File对象
PrintWriter pw = new PrintWriter(new FileWriter(file)); // 创建PrintWriter对象
pw.println(count); // 将计数器值写入文件
pw.close(); // 关闭PrintWriter
```
4. 在Servlet中使用以下代码来输出计数器的值:
```java
out.print("本站访问量:" + count); // 输出计数器的值
```
完整的Servlet代码如下:
```java
@WebServlet("/counter")
public class CounterServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=utf-8");
PrintWriter out = response.getWriter();
ServletContext application = getServletContext();
String filePath = application.getRealPath("/counter/count.txt");
File file = new File(filePath);
int count = 0;
if (file.exists()) {
BufferedReader br = new BufferedReader(new FileReader(file));
count = Integer.parseInt(br.readLine());
br.close();
}
count++;
PrintWriter pw = new PrintWriter(new FileWriter(file));
pw.println(count);
pw.close();
out.print("本站访问量:" + count);
}
}
```
在JSP页面中,可以使用以下代码来显示计数器的值:
```jsp
本站访问量:<%=application.getAttribute("count")%>
```
在JSP页面的`<body>`标签中添加以下代码来调用计数器Servlet:
```jsp
<jsp:include page="/counter"/>
```
在Web应用程序的`web.xml`文件中添加以下代码来初始化计数器的值:
```xml
<context-param>
<param-name>count</param-name>
<param-value>0</param-value>
</context-param>
```
在Web应用程序的`ServletContextListener`实现类中,可以使用以下代码来读取和更新计数器的值:
```java
public class MyServletContextListener implements ServletContextListener {
public void contextInitialized(ServletContextEvent event) {
ServletContext application = event.getServletContext();
String filePath = application.getRealPath("/counter/count.txt");
File file = new File(filePath);
int count = 0;
if (file.exists()) {
try {
BufferedReader br = new BufferedReader(new FileReader(file));
count = Integer.parseInt(br.readLine());
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
application.setAttribute("count", count);
}
public void contextDestroyed(ServletContextEvent event) {
ServletContext application = event.getServletContext();
String filePath = application.getRealPath("/counter/count.txt");
File file = new File(filePath);
int count = (Integer)application.getAttribute("count");
PrintWriter pw;
try {
pw = new PrintWriter(new FileWriter(file));
pw.println(count);
pw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在Web应用程序的`web.xml`文件中添加以下代码来注册`MyServletContextListener`:
```xml
<listener>
<listener-class>com.example.MyServletContextListener</listener-class>
</listener>
```
这样就可以使用文件存储方式来实现网站计数器的功能了。
阅读全文
相关推荐
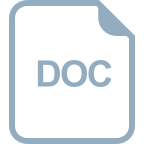
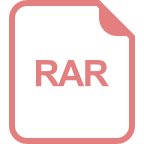
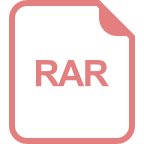
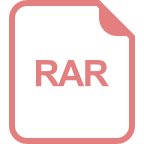
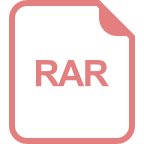
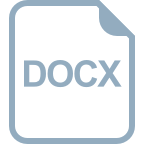
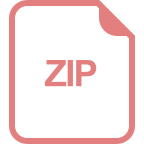
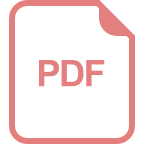
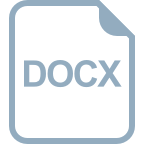
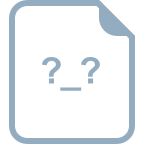
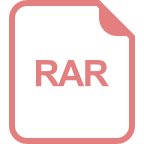
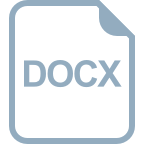
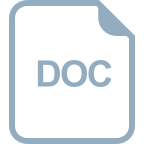
