如何在Python中定义和传递字典类型的参数?
时间: 2024-09-08 20:03:08 浏览: 26
在Python中,字典是一种可变容器模型,且可存储任意类型对象。字典的每个键值对用冒号 `:` 分割,每个对之间用逗号 `,` 分割,整个字典包括在花括号 `{}` 中。以下是定义和传递字典类型参数的步骤:
1. 定义字典:
字典可以通过花括号 `{}` 直接定义,也可以使用 `dict()` 函数创建。
```python
# 使用花括号定义字典
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# 使用dict()函数定义字典
my_dict = dict(name='Alice', age=25, city='New York')
```
2. 传递字典参数给函数:
字典可以作为参数传递给函数,函数内部可以通过键值对来访问字典中的数据。
```python
def print_user_info(user_info):
print(f"Name: {user_info['name']}, Age: {user_info['age']}, City: {user_info['city']}")
# 创建字典
user = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# 调用函数并传递字典参数
print_user_info(user)
```
3. 使用关键字参数传递:
在函数定义时可以设置默认参数值,调用时可以只传递需要修改的字典键值对。
```python
def create_user(name, age, city='Unknown'):
return {'name': name, 'age': age, 'city': city}
# 使用关键字参数调用函数
user_info = create_user(name='Alice', age=25)
print(user_info)
```
4. 使用**解包操作符:
如果字典以参数形式传递给函数,可以使用 `**` 操作符来解包字典。
```python
def print_user_info(**user_info):
for key, value in user_info.items():
print(f"{key}: {value}")
# 创建字典
user = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# 使用**操作符解包字典并传递给函数
print_user_info(**user)
```
相关推荐
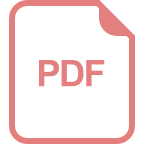
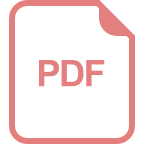
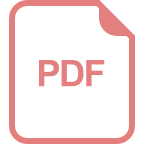














