spinbox 输出字母
时间: 2024-09-19 20:06:15 浏览: 21
SpinBox是Qt中的一种控件,它通常用于显示和编辑整数或小数值。如果你想让它输出字母而不是数字,你可以通过设置其`setAlignment()`属性以及连接特定信号到槽函数来实现。
首先,确保你的SpinBox已经创建并设置了初始值类型为`QLineEdit`,因为这样才能支持文本输入:
```cpp
QSpinBox *spinBox = new QSpinBox(this);
spinBox->setAlignment(Qt::AlignRight | Qt::AlignVCenter); // 设置文字居右对齐
spinBox->setMinimum(-26); // 如果你想限制字母范围,可以设定最小值为A的ASCII码减去1
spinBox->setMaximum(25); // 同理,设定最大值为Z的ASCII码
// 连接信号槽,当框内内容改变时处理字符输入
QObject::connect(spinBox, &QSpinBox::valueChanged, this, [spinBox](int value) {
QString letter = static_cast<char>(value + 'A'); // 将数字转换为大写字母(A对应0)
spinBox->setText(letter);
});
```
这样,当你在SpinBox上输入数字,它会自动转换成对应的字母。如果你需要支持更复杂的输入验证或者字母大小写的切换,你可能还需要添加额外的逻辑。
相关问题
SpinBox returnPressed
`returnPressed` 是 `QSpinBox` 类的一个信号,表示当用户在 `QSpinBox` 中按下 Enter 键时会触发该信号。你可以使用 `connect` 函数将该信号与一个槽函数连接起来,槽函数可以在用户按下 Enter 键时执行一些操作,例如更新界面或者进行计算等等。以下是一个使用 `returnPressed` 信号的例子:
```python
from PyQt5.QtWidgets import QApplication, QSpinBox, QVBoxLayout, QWidget
def on_spinbox_returnPressed():
print('Enter key pressed in spinbox')
app = QApplication([])
window = QWidget()
spinbox = QSpinBox()
spinbox.returnPressed.connect(on_spinbox_returnPressed)
layout = QVBoxLayout()
layout.addWidget(spinbox)
window.setLayout(layout)
window.show()
app.exec_()
```
在这个例子中,我们创建了一个 `QSpinBox` 控件,并将其 `returnPressed` 信号连接到名为 `on_spinbox_returnPressed` 的槽函数上。当用户在 `QSpinBox` 中按下 Enter 键时,`on_spinbox_returnPressed` 函数将会被调用,打印出一条消息。最后,我们将 `QSpinBox` 放置在一个垂直布局中,并显示窗口。
spinbox外观美化
spinbox是一个用户界面组件,通常用于让用户从一组预设选项中选择一个值,它看起来像一个小型的下拉列表。如果你想美化QSpinBox(在Qt库中常见),可以考虑以下几个方面:
1. **样式定制**:
- 使用`setStyleSheet()`方法改变其背景、边框、字体等样式属性,可以调整其外观颜色、圆角、边距等。
```python
qspinbox.setStyleSheet("QSpinBox {background-color: #f0f0f0; border-radius: 5px; padding: 5px}")
```
2. **主题应用**:
如果你的应用程序有统一的主题风格,你可以设置成对应的主题色,比如使用Qt提供的样式表。
3. **图标或图像**:
可以通过`setPixmap()`方法添加前缀或后缀图标的显示,提升交互体验。
4. **尺寸调整**:
调整`sizePolicy()`来控制spinbox占据的空间大小,使之与其他元素更协调。
```python
qspinbox.setSizePolicy(QSizePolicy.Fixed, QSizePolicy.Fixed)
```
阅读全文
相关推荐
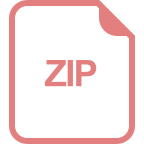
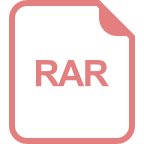
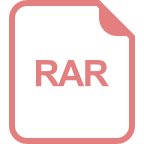













