用尾插法创建一个带头节点的c语言编程
时间: 2024-12-22 21:17:52 浏览: 9
在C语言中,使用尾插法创建一个带头节点的动态链表并不常见,因为链表通常不需要堆的概念。不过,如果你想要模拟类似归并排序的后序过程,可以将新元素插入到链表的末尾,我们可以创建一个简单的单向链表结构,并编写一个函数来实现这个功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* create_node(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = data;
newNode->next = NULL; // 初始化为NULL,表示这是尾部
return newNode;
}
// 尾插法添加元素到链表末尾
void insert_tail(Node** head, int data) {
Node* newNode = create_node(data);
if (*head == NULL) { // 如果链表为空,直接设置头结点
*head = newNode;
return;
}
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode; // 插入到链表尾部
}
// 打印链表
void print_list(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
insert_tail(&head, 5); // 添加元素5
insert_tail(&head, 2);
insert_tail(&head, 8);
print_list(head);
return 0;
}
```
在这个示例中,`insert_tail` 函数会将给定的值插入到链表的最后,而`main`函数展示了如何使用这个函数创建并打印一个简单的链表。
阅读全文
相关推荐


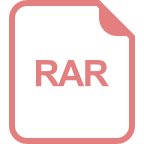











