Linux的C语言多线程编程中,如果有一个线程thread1正常运行,定时器根据信号量执行2ms时间间隔间隔的函数time_func,当定时器到期时,保存thread1的线程上下文挂起,当time_func运行结束后,恢复线程thread1,请给出具体详细的代码并说明。
时间: 2024-12-10 21:46:16 浏览: 17
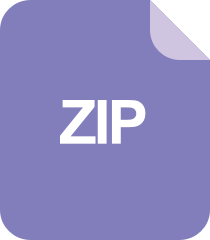
c语言多进程多线程编程
在Linux C语言中,使用POSIX线程库(pthreads)可以实现这样的多线程场景。下面是一个简单的示例,使用`pthread_cond_timedwait()`和`pthread_mutex_lock()`/`pthread_mutex_unlock()`以及`sigaction()`处理定时器和线程切换:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <pthread.h>
#define TIMER_INTERVAL 2000 // 2 ms
// 信号量
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
// 定义线程函数
void *time_func(void *arg) {
while (1) {
pthread_mutex_lock(&mutex);
printf("Time Func running...\n");
// 假设这个函数执行需要一定时间,这里省略了实际操作
sleep(TIMER_INTERVAL / 1000); // 模拟2ms执行
pthread_cond_signal(&cond); // 任务完成,唤醒主线程
pthread_mutex_unlock(&mutex);
}
return NULL;
}
void *thread1_func(void *arg) {
int status;
while (1) {
// 线程1运行...
// 当需要切换到time_func时,设置条件变量
pthread_mutex_lock(&mutex);
printf("Thread1 suspended by timer\n");
pthread_cond_wait(&cond, &mutex); // 挂起直到time_func结束
pthread_mutex_unlock(&mutex);
// 线程1继续运行...
}
return NULL;
}
int main() {
pthread_t thread1, time_thread;
if (pthread_create(&time_thread, NULL, time_func, NULL)) {
perror("Creating time thread failed");
exit(EXIT_FAILURE);
}
if (pthread_create(&thread1, NULL, thread1_func, NULL)) {
perror("Creating thread1 failed");
exit(EXIT_FAILURE);
}
// 主线程等待并监控其他线程
pthread_join(thread1, &status);
if (WIFEXITED(status) && WEXITSTATUS(status) == 0)
printf("Thread1 ended with success.\n");
else if (WIFSIGNALED(status))
printf("Thread1 terminated by signal %d.\n", WTERMSIG(status));
pthread_join(time_thread, &status);
if (WIFEXITED(status) && WEXITSTATUS(status) == 0)
printf("Time thread ended with success.\n");
else if (WIFSIGNALED(status))
printf("Time thread terminated by signal %d.\n", WTERMSIG(status));
return 0;
}
```
在这个例子中,`time_func`是一个无限循环的线程,它会定期执行并在`timer_interval`时间后唤醒主线程。`thread1_func`在线程1运行期间,当收到信号时暂停并等待`time_func`完成。
注意:这个示例中没有直接使用`signal()`处理定时器,而是通过`sleep()`模拟定时器。在实际应用中,通常会使用`alarm()`、`itimer_set()`或更专业的库如librt的定时器。此外,为了精确控制线程睡眠和唤醒,可能还需要调整`sleep()`的时间,并在`time_func`里处理定时事件的具体逻辑。
阅读全文
相关推荐
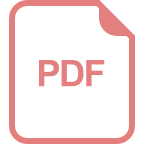
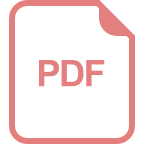
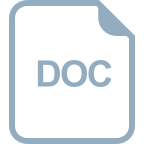
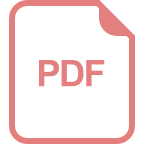
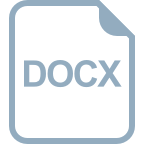
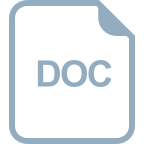
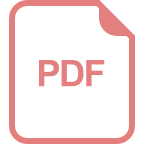
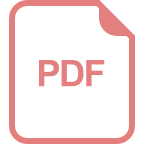
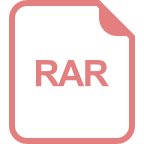
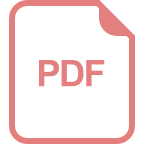
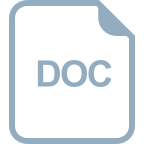
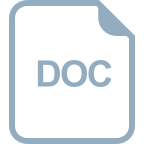