使用c++编写局域网考核程序代码
时间: 2024-12-09 09:29:12 浏览: 16
编写一个简单的局域网考核程序代码涉及到网络编程和数据结构等知识,以下是一个基本的例子,展示了如何在C++中创建一个简单的学生信息服务器,允许客户端发送学生的ID并获取其成绩:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
class Student {
public:
std::string id;
int score;
// 构造函数、getter和setter省略
};
// 定义服务器端的处理函数
void handle_client(tcp::socket socket, std::map<std::string, Student>& student_map) {
tcp::no_delay(socket); // 关闭 Nagle 算法提高效率
std::string request;
std::getline(socket, request); // 接收请求(学生ID)
auto it = student_map.find(request);
if (it != student_map.end()) {
std::cout << "Sending score for student " << request << ": " << it->second.score << "\n";
std::string response = "Score: " + std::to_string(it->second.score);
write(socket, response.begin(), response.end());
} else {
write(socket, "Student not found.\n".begin(), "Student not found.\n".end());
}
}
int main() {
try {
boost::asio::io_context io_context;
tcp::acceptor acceptor(io_context, tcp::endpoint(tcp::v4(), 8000)); // 设置监听端口
std::map<std::string, Student> student_db; // 存储学生信息
for (;;) { // 永久循环等待连接
tcp::socket socket(io_context);
acceptor.accept(socket);
std::thread client_thread(handle_client, std::move(socket), std::ref(student_db));
client_thread.detach(); // 非阻塞模式
}
}
catch (std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
这个程序通过`boost::asio`库建立了一个TCP服务器,监听8000端口。当收到客户端的请求(学生ID)后,它会从存储的学生数据库中查找对应的成绩,并将结果返回给客户端。
阅读全文
相关推荐
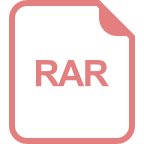








