写一个用python实现支持向量机分类的代码并有相应的可视化程序
时间: 2024-10-12 08:15:56 浏览: 13
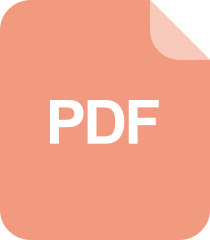
Python 支持向量机分类器的实现

在Python中,我们可以使用Scikit-Learn库中的SVM(Support Vector Machine)模块来实现分类。这里是一个简单的示例,使用Iris数据集,并包括可视化支持向量机决策边界的过程。首先确保你已经安装了所需的库,如果没有,可以通过pip安装:
```python
# 导入所需库
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
import matplotlib.pyplot as plt
import seaborn as sns
# 加载Iris数据集
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 数据预处理:标准化特征值
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.2, random_state=42)
# 使用SVC创建模型
svm_model = SVC(kernel='linear') # 这里选择线性核,如果是非线性问题可以选择 'rbf', 'poly' 等
svm_model.fit(X_train, y_train)
# 预测测试集
y_pred = svm_model.predict(X_test)
# 可视化决策边界
def plot_decision_boundary(model, X, y):
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
xx, yy = np.meshgrid(np.linspace(x_min, x_max, 100),
np.linspace(y_min, y_max, 100))
Z = model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.8)
plt.scatter(X[y == 0, 0], X[y == 0, 1], color='red', label='Class 0')
plt.scatter(X[y == 1, 0], X[y == 1, 1], color='blue', label='Class 1')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.legend()
plot_decision_boundary(svm_model, X_scaled, y)
# 显示图形
plt.show()
# 结果评估
from sklearn.metrics import classification_report, confusion_matrix
print("Classification Report:")
print(classification_report(y_test, y_pred))
print("Confusion Matrix:")
print(confusion_matrix(y_test, y_pred))
```
在这个例子中,我们创建了一个线性支持向量机模型,然后绘制了数据点及其预测的决策边界。最后,我们展示了分类报告和混淆矩阵来评估模型性能。
阅读全文
相关推荐
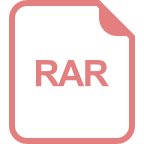
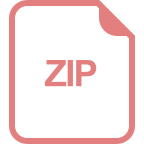
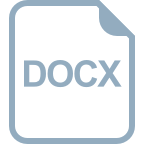
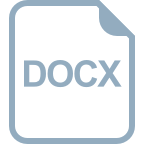
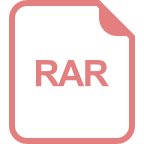
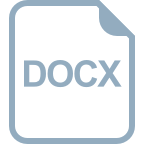
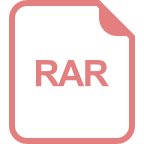
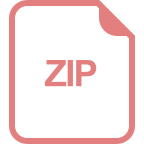
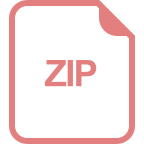
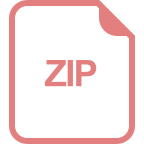
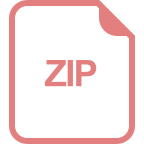
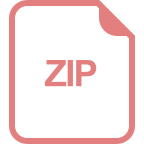
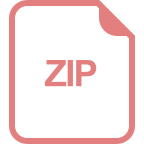
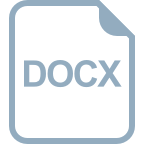
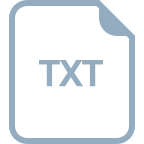
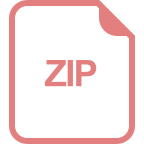
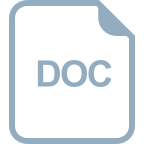
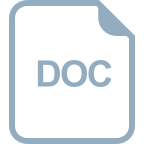