用C语言指针实现两个字符串的公共子串的输出。
时间: 2024-10-29 13:10:43 浏览: 35
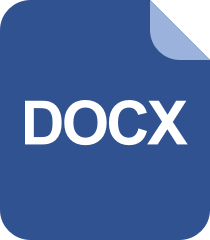
C语言基础面试题(03-字符串函数).docx
在C语言中,我们可以使用指针来处理字符串,并通过动态内存分配找到两个字符串之间的公共子串。这里提供一种基本的方法:
1. 首先,我们需要创建两个指向字符数组的指针,分别指向我们要比较的两个字符串。
```c
char *str1 = "字符串1";
char *str2 = "另一个字符串";
```
2. 然后,我们计算每个字符串的长度,通常会遍历到空字符 '\0' 所在的位置。
```c
int len1 = strlen(str1);
int len2 = strlen(str2);
```
3. 定义一个变量 `max_len` 存储最大可能的公共子串长度,初始化为较小的字符串长度。这是因为最短的字符串不可能有比它本身更长的公共子串。
```c
int max_len = min(len1, len2);
```
4. 使用循环遍历两个字符串的范围,从头开始逐个字符对比,如果找到相同的字符,则递减 `max_len` 并更新公共子串起始位置 `start_index`。
```c
int start_index = 0;
while (max_len > 0) {
if (str1[start_index] == str2[start_index]) {
start_index++;
max_len--;
} else {
start_index = 0; // 如果字符不同,跳回开头继续查找
}
}
```
5. 检查 `start_index` 是否小于或等于 `len1` 和 `len2`,因为有可能找到了完全相同的字符串,此时 `start_index` 就是公共子串的实际长度。
6. 最后,根据 `start_index` 和原始字符串,可以动态地分配内存并打印出公共子串。
```c
if (start_index > 0) {
char *common_substring = malloc(start_index + 1); // +1 for the null terminator
strncpy(common_substring, str1, start_index);
common_substring[start_index] = '\0'; // 添加空字符结束字符串
printf("公共子串: %s\n", common_substring);
free(common_substring);
} else {
printf("没有公共子串.\n");
}
```
阅读全文
相关推荐
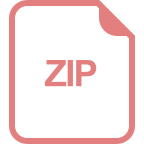
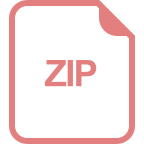



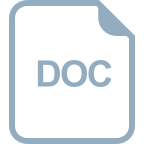
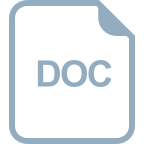
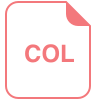


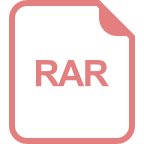
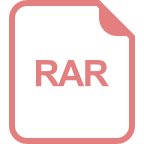
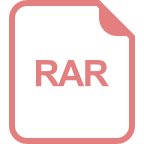
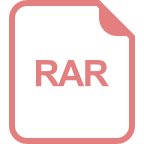
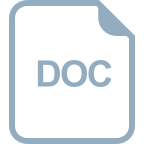
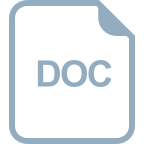
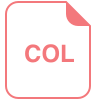
