c#直线与圆是否相交
时间: 2024-10-23 09:17:48 浏览: 25
在C#中,判断直线与圆是否相交通常需要一些几何计算。一种常见的方法是通过向量和点到圆心的距离来确定。以下是基本步骤:
1. **获取直线和圆的基本信息**:
- 直线由两个点定义,可以用它们的斜率(m = (y2-y1) / (x2-x1))和一个截距(b)表示,或使用参数方程。
- 圆则由圆心坐标(xc, yc)和半径r给出。
2. **计算距离**:
- 计算直线上的任意一点P(x, y)到圆心的距离d = Math.Sqrt((x-xc)^2 + (y-yc)^2),如果这个距离小于等于半径,则说明直线与圆相交。
3. **特殊情况处理**:
- 如果直线的斜率不存在(垂直线),可以特殊处理,例如当直线穿过圆心时认为是完全相交;
- 如果直线平行于x轴(斜率为0),只需比较圆心到直线的水平距离,若小于半径也是相交;
4. **计算交点**:
- 如果需要找到具体的交点,可以设置另一个点Q在直线上的某个位置,然后解方程组找出圆上满足条件的交点。
```csharp
bool IntersectCircleLine(Point circleCenter, double circleRadius, Point lineStart, Point lineEnd)
{
// ... 实现上述步骤
}
```
相关问题
c# 判断直线和矩形相交
判断直线和矩形是否相交,可以分为两个步骤:
1. 判断直线是否与矩形相交
2. 如果相交,判断交点是否在矩形内部
下面是一个示例代码实现:
```csharp
public bool IsLineIntersectRectangle(PointF p1, PointF p2, RectangleF rect)
{
// 判断直线是否与矩形相交
if (!rect.IntersectsWith(new RectangleF(Math.Min(p1.X, p2.X), Math.Min(p1.Y, p2.Y), Math.Abs(p1.X - p2.X), Math.Abs(p1.Y - p2.Y))))
{
return false;
}
// 计算直线的斜率和截距
float k = (p2.Y - p1.Y) / (p2.X - p1.X);
float b = p1.Y - k * p1.X;
// 判断交点是否在矩形内部
PointF[] corners = new PointF[] { new PointF(rect.Left, rect.Top), new PointF(rect.Right, rect.Top), new PointF(rect.Right, rect.Bottom), new PointF(rect.Left, rect.Bottom) };
for (int i = 0; i < corners.Length; i++)
{
PointF corner1 = corners[i];
PointF corner2 = corners[(i + 1) % corners.Length];
// 计算矩形边的斜率和截距
float k2 = (corner2.Y - corner1.Y) / (corner2.X - corner1.X);
float b2 = corner1.Y - k2 * corner1.X;
// 计算交点
float x = (b2 - b) / (k - k2);
float y = k * x + b;
// 判断交点是否在矩形内部
if (x >= rect.Left && x <= rect.Right && y >= rect.Top && y <= rect.Bottom)
{
return true;
}
}
return false;
}
```
其中,`p1` 和 `p2` 分别表示直线的起点和终点,`rect` 表示矩形。函数返回 `true` 表示直线和矩形相交,返回 `false` 表示不相交。
用C# 写一个2直线求相交点的程序
在 C# 中,你可以通过创建一个简单的数学类,利用向量运算来计算两条直线的交点。假设两条直线可以用以下形式表示:
线A:(x1, y1) + t * (dx, dy)
线B:(x2, y2) + s * (dx2, dy2)
其中 (x1, y1), (x2, y2) 是两条直线的两个端点,(dx, dy), (dx2, dy2) 分别是两条直线的方向向量。
下面是一个基础的示例代码:
```csharp
using System;
public class Vector
{
public double X { get; set; }
public double Y { get; set; }
public Vector(double x, double y)
{
X = x;
Y = y;
}
// 向量加法
public static Vector operator +(Vector a, Vector b)
{
return new Vector(a.X + b.X, a.Y + b.Y);
}
// 向量减法
public static Vector operator -(Vector a, Vector b)
{
return new Vector(a.X - b.X, a.Y - b.Y);
}
// 点积
public static double DotProduct(Vector a, Vector b)
{
return a.X * b.X + a.Y * b.Y;
}
}
public class Line
{
private Vector _start;
private Vector _direction;
public Line(Vector start, Vector direction)
{
_start = start;
_direction = direction;
}
public Vector GetIntersection(Line other)
{
if (_direction.Equals(other._direction)) // 如果两条直线平行
return null;
// 计算交叉率
double crossRatio = ((_start - other._start).DotProduct(other._direction) / _direction.DotProduct(other._direction));
// 检查交叉率是否在0到1范围内
if (crossRatio < 0 || crossRatio > 1)
return null;
return _start + _direction * crossRatio;
}
}
class Program
{
static void Main(string[] args)
{
Vector pointA = new Vector(0, 0);
Vector directionA = new Vector(1, 1); // 直线A斜率为1,向上右方向
Vector pointB = new Vector(4, 4); // 直线A的一个端点
Vector pointC = new Vector(5, 0);
Vector directionC = new Vector(0, 1); // 直线B斜率为0,垂直向下
Line lineA = new Line(pointA, directionA);
Line lineB = new Line(pointC, directionC);
Vector intersection = lineA.GetIntersection(lineB);
if (intersection != null)
Console.WriteLine($"Lines intersect at ({intersection.X}, {intersection.Y})");
else
Console.WriteLine("Lines do not intersect.");
}
}
```
这个程序首先定义了一个 `Vector` 类,用于处理二维向量。然后创建了 `Line` 类,其中包含计算交点的方法。在 `Main` 函数中,我们实例化了两条直线并调用 `GetIntersection` 方法检查它们是否相交。
阅读全文
相关推荐
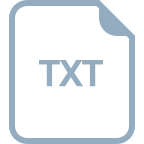
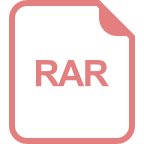
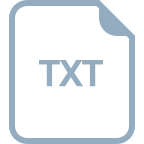
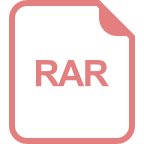
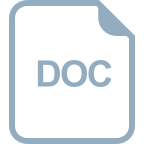
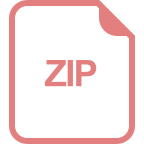
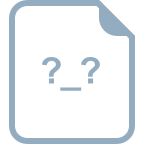
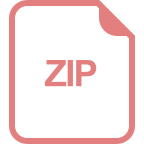
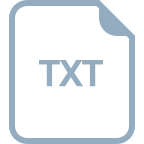
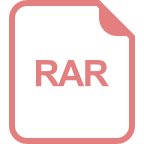
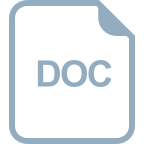


