用c语言创建一个单链表,要求创建链表能够输入元素(用键盘输入)并保持有序,插入元素(按位置插入)并保持有序,查找,删除(按位置删除)且能够删除相同的值
时间: 2024-09-27 14:08:00 浏览: 31
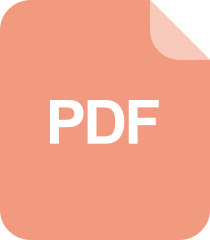
C语言 数据结构双向链表简单实例
在C语言中,我们可以使用结构体和指针来创建一个简单的单链表,并实现添加、查找和删除功能。首先,我们需要定义一个链表节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建链表
Node* createList() {
return NULL;
}
// 添加元素到链表(按顺序)
void insert(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (*head == NULL) {
newNode->data = value;
newNode->next = NULL;
*head = newNode;
} else if (value <= (*head)->data) {
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
}
}
// 查找元素
Node* search(Node* head, int value) {
Node* current = head;
while (current != NULL) {
if (current->data == value)
return current;
current = current->next;
}
return NULL;
}
// 删除元素(按位置)
void delete(Node** head, int position) {
if (*head == NULL || position <= 0)
return;
Node* temp = *head;
if (position == 1) {
*head = (*head)->next;
free(temp);
return;
}
for (int i = 1; temp != NULL && i < position - 1; i++) {
temp = temp->next;
}
if (temp != NULL) {
temp->next = temp->next->next;
free(temp->next);
}
}
// 打印链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
Node* head = createList();
int choice, value, position;
while (1) {
printf("\n1. Add element\n2. Search element\n3. Delete element by position\n4. Print list\n5. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter value to add: ");
scanf("%d", &value);
insert(&head, value);
break;
case 2:
printf("Enter value to find: ");
scanf("%d", &value);
Node* found = search(head, value);
if (found)
printf("Found at position %d.\n", position + 1); // 位置从1开始计数
else
printf("Element not found.\n");
break;
case 3:
printf("Enter position to delete (1-based): ");
scanf("%d", &position);
delete(&head, position);
break;
case 4:
printList(head);
break;
case 5:
exit(0);
default:
printf("Invalid choice. Try again.\n");
}
}
return 0;
}
```
这个程序实现了基本的链表操作,包括添加(add)、查找(search)、删除(delete)以及打印(print)。注意,因为是在命令行交互式环境中运行,所以没有涉及用户输入元素的结束条件。
阅读全文
相关推荐
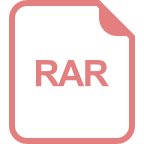
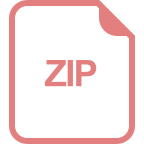
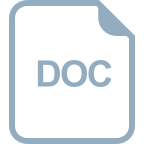
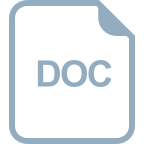
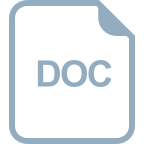
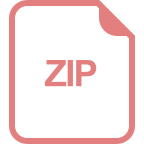
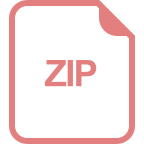
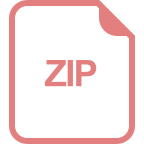