两人对弈的五子棋游戏java代码
时间: 2024-01-02 10:00:49 浏览: 27
五子棋是一种古老的策略性棋类游戏,通常由两个玩家轮流下棋。下面是一个简单的用Java实现的两人对弈的五子棋游戏代码:
```
import java.util.Scanner;
public class GomokuGame {
private char[][] board;
private int currentPlayer;
private boolean isGameOver;
public GomokuGame() {
board = new char[15][15];
currentPlayer = 1;
isGameOver = false;
}
public void playGame() {
Scanner scanner = new Scanner(System.in);
while (!isGameOver) {
System.out.println("玩家 " + currentPlayer + " 的回合");
System.out.print("请输入行号和列号(以空格分隔):");
int row = scanner.nextInt();
int col = scanner.nextInt();
if (isValidMove(row, col)) {
makeMove(row, col);
printBoard();
if (checkWin(row, col)) {
isGameOver = true;
System.out.println("玩家 " + currentPlayer + " 获胜!");
} else if (isBoardFull()) {
isGameOver = true;
System.out.println("平局!");
}
currentPlayer = (currentPlayer == 1) ? 2 : 1; // 切换玩家
} else {
System.out.println("无效的移动,请重新输入");
}
}
scanner.close();
}
public boolean isValidMove(int row, int col) {
return (row >= 0 && row < 15 && col >= 0 && col < 15 && board[row][col] == '\u0000');
}
public void makeMove(int row, int col) {
board[row][col] = (currentPlayer == 1) ? 'X' : 'O';
}
public boolean checkWin(int row, int col) {
char symbol = (currentPlayer == 1) ? 'X' : 'O';
// 检查横向是否有五子连线
int count = 0;
for (int i = Math.max(0, col - 4); i <= Math.min(14, col + 4); i++) {
if (board[row][i] == symbol) {
count++;
if (count == 5) {
return true;
}
} else {
count = 0;
}
}
// 检查纵向是否有五子连线
count = 0;
for (int i = Math.max(0, row - 4); i <= Math.min(14, row + 4); i++) {
if (board[i][col] == symbol) {
count++;
if (count == 5) {
return true;
}
} else {
count = 0;
}
}
// 检查左上到右下斜线是否有五子连线
count = 0;
for (int i = Math.max(row - 4, 0), j = Math.max(col - 4, 0); i <= Math.min(row + 4, 14) && j <= Math.min(col + 4, 14); i++, j++) {
if (board[i][j] == symbol) {
count++;
if (count == 5) {
return true;
}
} else {
count = 0;
}
}
// 检查左下到右上斜线是否有五子连线
count = 0;
for (int i = Math.min(row + 4, 14), j = Math.max(col - 4, 0); i >= Math.max(row - 4, 0) && j <= Math.min(col + 4, 14); i--, j++) {
if (board[i][j] == symbol) {
count++;
if (count == 5) {
return true;
}
} else {
count = 0;
}
}
return false;
}
public boolean isBoardFull() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
if (board[i][j] == '\u0000') {
return false;
}
}
}
return true;
}
public void printBoard() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
System.out.print(board[i][j] == '\u0000' ? '-' : board[i][j]);
}
System.out.println();
}
}
public static void main(String[] args) {
GomokuGame game = new GomokuGame();
game.playGame();
}
}
```
以上就是实现两人对弈的五子棋游戏的简单Java代码。玩家可以依次输入行号和列号来下棋,当有一方连成五子时,游戏结束并宣布获胜者。如果棋盘已满而无法继续下棋,则宣布平局。
相关推荐
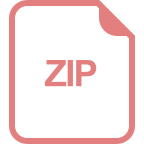
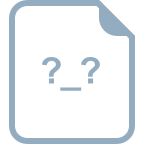














