pandas knn
时间: 2023-10-28 09:57:40 浏览: 62
Pandas is a popular Python library for data manipulation and analysis. It provides various functionalities for working with structured data, such as data frames. However, Pandas does not have an implementation of k-nearest neighbors (KNN) algorithm built-in.
To perform KNN using Pandas, you would typically use other libraries that provide implementation of the KNN algorithm, such as scikit-learn. Scikit-learn is a widely-used machine learning library in Python and it has a comprehensive set of algorithms, including KNN.
Here's an example of using KNN with Pandas and scikit-learn:
```python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
# Load data into a Pandas DataFrame
data = pd.read_csv('your_data.csv')
# Separate features and target variable
X = data.drop('target', axis=1)
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Create a KNN classifier with k=3
knn = KNeighborsClassifier(n_neighbors=3)
# Train the classifier
knn.fit(X_train, y_train)
# Predict the target variable for the test set
y_pred = knn.predict(X_test)
# Evaluate the model's performance
accuracy = knn.score(X_test, y_test)
print(f"Accuracy: {accuracy}")
```
Make sure to replace `'your_data.csv'` with the path to your actual data file. This code assumes that your data is in a CSV file format and the target variable column is named `'target'`.
相关推荐
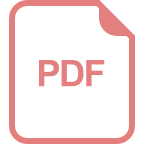
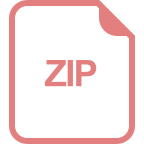














