c++string应用
时间: 2023-10-22 10:06:07 浏览: 108
C语言中的字符串是由字符数组组成的,以null字符('\0')作为结尾。C语言提供了一系列的库函数来处理字符串,如字符串的复制、连接、比较等操作。
下面是一些常用的C语言字符串函数:
1. strlen()函数:用于计算字符串的长度(不包括结尾的null字符)。
2. strcpy()函数:用于将一个字符串复制到另一个字符串。
3. strcat()函数:用于将一个字符串连接到另一个字符串的末尾。
4. strcmp()函数:用于比较两个字符串的大小,返回一个整数值。
5. strchr()函数:用于在字符串中查找指定字符的第一次出现。
6. strstr()函数:用于在字符串中查找指定子串的第一次出现。
以下是一个示例程序,演示了如何使用这些字符串函数:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "Hello";
char str2[100] = "World";
char str3[100];
// 使用strlen()函数计算字符串的长度
printf("Length of str1: %d\n", strlen(str1));
// 使用strcpy()函数复制字符串
strcpy(str3, str1);
printf("str3 after strcpy(): %s\n", str3);
// 使用strcat()函数连接字符串
strcat(str3, " ");
strcat(str3, str2);
printf("str3 after strcat(): %s\n", str3);
// 使用strcmp()函数比较字符串
int result = strcmp(str1, str2);
if (result < 0) {
printf("str1 is less than str2\n");
} else if (result > 0) {
printf("str1 is greater than str2\n");
} else {
printf("str1 is equal to str2\n");
}
// 使用strchr()函数查找字符
char* p = strchr(str3, 'o');
if (p != NULL) {
printf("First occurrence of 'o' in str3 is at index %d\n", p - str3);
}
// 使用strstr()函数查找子串
p = strstr(str3, "World");
if (p != NULL) {
printf("First occurrence of 'World' in str3 is at index %d\n", p - str3);
}
return 0;
}
```
阅读全文
相关推荐







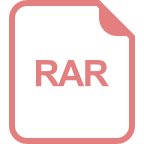

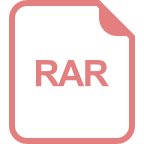







