编写代码,使得wonform在窗体2中的textbox里添加内容,点击窗体2中的按钮,能够将在窗体2中输入的内容传递到窗体1的textbox中
时间: 2024-09-24 15:26:59 浏览: 31
为了实现这种功能,你需要在两个窗体(Form1和Form2)之间建立一些通信机制。这里提供一种常见的解决方案——使用事件委托和公共方法。首先,在Form1中声明一个事件,然后在Form2中引发这个事件并将输入的数据携带过去。
**Form1.cs (接收窗体)**
```csharp
using System;
using System.Windows.Forms;
public partial class Form1 : Form
{
public event EventHandler<StringEventArgs> DataReceived;
public Form1()
{
InitializeComponent();
}
private void btnReceive_Click(object sender, EventArgs e)
{
if (DataReceived != null)
DataReceived(this, new StringEventArgs(textBoxInput.Text));
}
}
```
**Form2.cs (发送窗体)**
```csharp
using System;
using System.Windows.Forms;
public partial class Form2 : Form
{
public delegate void StringEventArgs(string data);
public event StringEventArgs SendData;
public Form2()
{
InitializeComponent();
buttonSend.Click += ButtonSend_Click;
}
private void ButtonSend_Click(object sender, EventArgs e)
{
if (SendData != null)
SendData(textBoxToSend.Text);
Close(); // 关闭Form2,防止无限循环
}
}
// 使用事件委托的方式在Form1中处理数据
public class Program
{
static void Main()
{
Application.Run(new Form1());
}
}
```
在上述代码中,Form2的`ButtonSend_Click`事件触发时,会调用`SendData`事件,并传递文本框`textBoxToSend`的内容。Form1捕获到这个事件后,在`btnReceive_Click`中处理并显示数据。
要测试这个功能,你需要在Form1的TextBox中绑接`DataReceived`事件,例如:
```csharp
private void Form1_Load(object sender, EventArgs e)
{
DataReceived += OnDataReceived;
}
private void OnDataReceived(object sender, StringEventArgs e)
{
textBoxInput.Text = e.Data;
}
```
这将实现在Form2的按钮点击后,窗体1的TextBox得到新内容的效果。
阅读全文
相关推荐
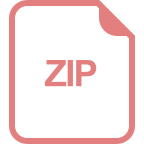
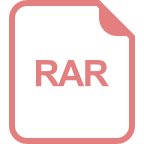
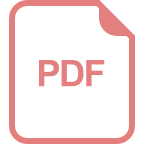
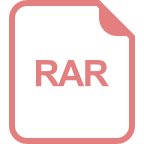
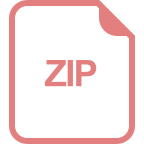
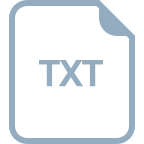
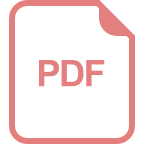
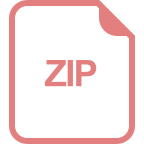
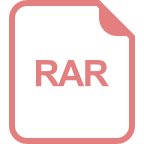
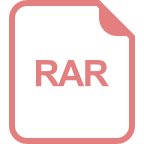
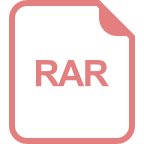
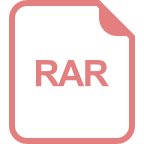
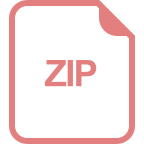
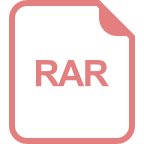
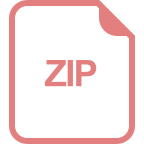
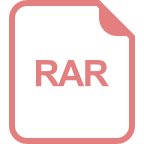
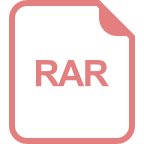
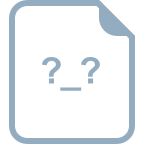