pyqt5连接sqlite数据库
时间: 2024-09-05 22:01:48 浏览: 56
PyQt5是一个跨平台的GUI工具包,它允许你使用Python语言创建窗口应用程序,而SQLite是一个轻量级的数据库引擎,广泛用于嵌入式设备和移动应用中。在PyQt5中连接SQLite数据库主要涉及使用Python的标准SQLite库,因为PyQt5本身并不提供数据库连接功能。
以下是使用PyQt5连接SQLite数据库的基本步骤:
1. 安装PyQt5和SQLite库(如果尚未安装):
```
pip install PyQt5
```
2. 在Python脚本中导入必要的模块:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget, QPushButton
import sqlite3
```
3. 创建数据库连接和游标对象:
```python
def connect_db():
conn = sqlite3.connect('example.db') # 连接到数据库,如果不存在则创建
return conn
```
4. 创建数据库表(如果尚未存在):
```python
def create_table():
conn = connect_db()
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS items
(id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL)''')
conn.commit()
conn.close()
```
5. 在PyQt5应用程序中使用连接和游标对象:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('PyQt5 SQLite Example')
self.setGeometry(100, 100, 400, 300)
# 创建UI组件,例如按钮用于执行数据库操作
self.button = QPushButton('Add Item', self)
self.button.clicked.connect(self.add_item)
layout = QVBoxLayout()
layout.addWidget(self.button)
container = QWidget()
container.setLayout(layout)
self.setCentralWidget(container)
create_table() # 确保数据库表已经创建
def add_item(self):
conn = connect_db()
cursor = conn.cursor()
cursor.execute('INSERT INTO items (name) VALUES (?)', ('Sample Item',))
conn.commit()
conn.close()
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
以上示例展示了如何在PyQt5应用程序中建立对SQLite数据库的连接,并进行基本的数据库操作。这里定义了一个简单的窗口,其中包含一个按钮,当点击按钮时,会向SQLite数据库的items表中插入一条记录。
阅读全文
相关推荐
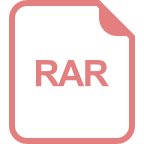
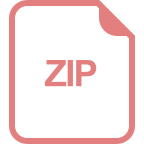



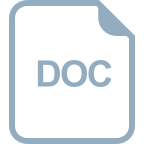



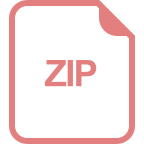
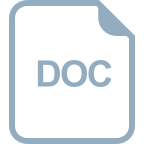
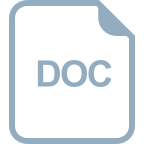
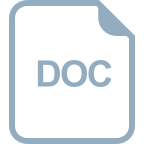




