httpclient get 参数
时间: 2023-12-09 09:05:52 浏览: 187
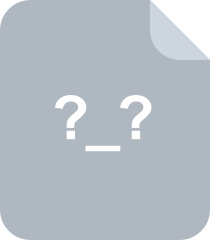
httpclient用法,发送get和post请求,设置header

HttpClient是一个开源的HTTP客户端库,它支持HTTP协议的客户端编程。在使用HttpClient进行GET请求时,需要传入参数,这些参数可以通过URI对象或者字符串直接拼接的方式传入。其中,URI对象可以通过URIBuilder类进行构建,而字符串直接拼接的方式则需要将参数以键值对的形式拼接在URL后面,多个参数之间用“&”符号连接。例如,引用中的代码使用了URIBuilder类构建了一个URI对象,并通过setParameter方法设置了两个参数“rn”和“list”。而引用中的代码则是使用字符串直接拼接的方式传入了一个参数。
阅读全文
相关推荐
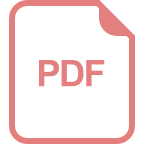
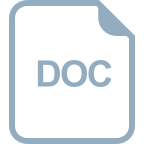
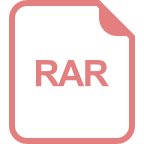
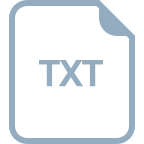
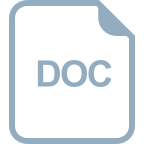
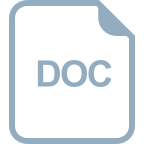








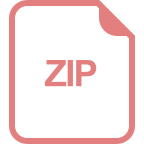