Json转化为实体类,Device类中有 private List<CarMileage> carMileageList = new ArrayList<>(); CarMileage中有 private List<MileBill> mileBillList = new ArrayList<>(); 如何将Json转换为List<Device>,同时保证所有属性都赋值正确
时间: 2024-01-01 12:35:26 浏览: 86
要将 JSON 转换为 List<Device>,您可以使用 JSON 库(如 Jackson、Gson 等)来执行转换。首先,您需要创建适合的实体类结构来表示 JSON 的结构。以下是一个示例:
```java
public class Device {
private List<CarMileage> carMileageList = new ArrayList<>();
// 其他属性和方法
// Getter 和 Setter 方法
}
public class CarMileage {
private List<MileBill> mileBillList = new ArrayList<>();
// 其他属性和方法
// Getter 和 Setter 方法
}
public class MileBill {
// MileBill 的属性
// Getter 和 Setter 方法
}
```
接下来,您可以使用 JSON 库来执行转换。以下是使用 Jackson 库的示例代码:
```java
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
// JSON 字符串
String json = "your_json_string";
// 创建 ObjectMapper 对象
ObjectMapper objectMapper = new ObjectMapper();
// 将 JSON 转换为 List<Device>
List<Device> deviceList = objectMapper.readValue(json, new TypeReference<List<Device>>() {});
// 遍历设备列表
for (Device device : deviceList) {
// 打印设备信息
System.out.println(device);
}
```
请注意,上述代码中的 "your_json_string" 需要替换为您实际的 JSON 字符串。此外,您需要根据实际的 JSON 结构和属性名称,在实体类中添加适当的属性和方法。
使用其他 JSON 库(如 Gson)也可以执行类似的转换操作。具体使用哪个库取决于您的项目需求和偏好。
阅读全文
相关推荐
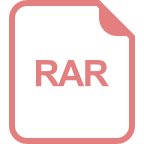


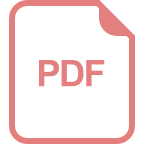
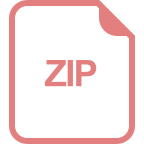
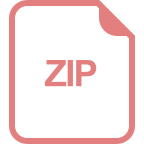
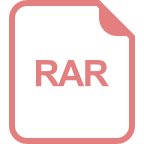
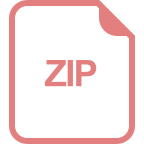
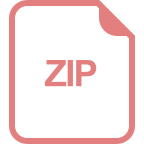
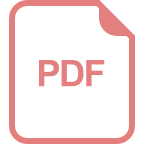
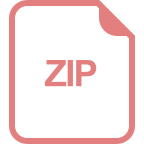
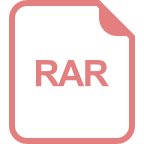
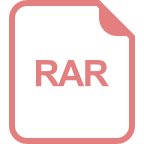
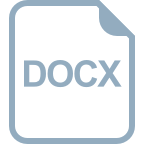
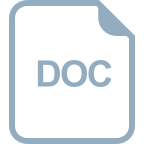
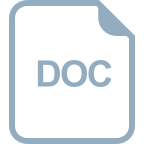
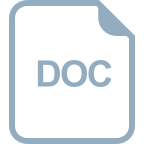