a算法解决八数码问题java
时间: 2023-10-18 15:03:25 浏览: 55
八数码问题是一个经典的数学问题,它的目标是通过重新排列一个3x3的数字方格,使得数字按照从小到大的顺序排列(0代表空格)。A*算法是一种常用的解决八数码问题的启发式搜索算法。
在Java中,我们可以使用A*算法来解决八数码问题。首先,我们需要定义一个表示数字方格的数据结构,可以使用二维数组或者自定义的类来表示。每个数字方格都有一个状态,表示数字方格的当前排列。另外,还需要定义一个优先队列来存储待拓展的数字方格状态。
A*算法的核心思想是采用估价函数来评估每个待拓展状态的优先级,选择优先级最高的状态进行拓展。在八数码问题中,我们可以使用曼哈顿距离作为估价函数,即计算每个数字在当前位置与目标位置之间的曼哈顿距离之和。这个估价函数可以帮助我们评估当前状态离目标状态的距离,从而选择移动最小的状态来进行拓展。
具体实现时,我们可以使用一个while循环来不断从优先队列中取出优先级最高的状态进行拓展。在每次拓展中,我们可以先计算当前状态的哈希值,用于判断是否已经达到解决目标。然后,我们可以根据当前状态的邻居状态和移动的代价(即曼哈顿距离)来计算新的状态,并将其加入优先队列中。
当取出的状态为目标状态时,我们可以通过回溯的方式找到从初始状态到目标状态的具体解决步骤。最后,我们可以输出这些步骤,即表示八数码问题的解决方案。
总之,A*算法是一种高效解决八数码问题的启发式搜索算法,通过合理选择估价函数和优先队列的实现,可以在较短的时间内找到八数码问题的解决方案。在Java中,可以通过定义合适的数据结构和实现算法的细节来完成这个任务。
相关问题
a*算法解决八数码问题java
A*算法是一种启发式搜索算法,它利用启发函数(heuristic function)来估计从当前状态到目标状态的距离,以选择最优的路径。在八数码问题中,A*算法可以通过解析8个数字和一个空格的排列状态,来完成拼图问题。
A*算法需要记录每个状态的成本值和路径,同时需要建立一个开放列表(open list)和一个关闭列表(closed list)来记录搜索过程中的状态。其中,开放列表存储待扩展的状态,关闭列表存储已经扩展的状态。
对于八数码问题,启发函数可以选择已经放置正确的数字数量,或者每个数字离它正确的位置的距离总和等作为估价函数。A*算法以估计值加上已经扩展的成本值得到目标状态的估计总成本,按照成本值从小到大优先扩展。
在Java中实现A*算法解决八数码问题,可以采用BFS(Breadth-First-Search)搜索遍历算法来构建搜索树,用PriorityQueue数据结构来维护开放列表,用HashSet数据结构来维护关闭列表,以及一个HashMap来存储每个节点的路径和成本值。对于每个节点,需要判断它是否能够到达目标状态,如果能够到达,则通过HashMap从目标节点追踪回到起始节点,得到解决八数码问题的路径。
八数码问题a*算法java
以下是使用A*算法解决八数码问题的Java代码示例:
```java
import java.util.*;
public class EightPuzzle {
private static final int[][] GOAL = {{1, 2, 3}, {4, 5, 6}, {7, 8, 0}}; // 目标状态
private static final int[][] DIRS = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}}; // 上下左右四个方向
private static final int N = 3; // 棋盘大小
private static final int MAX_STATE = 1000000; // 最大状态数
private static int[][] start = new int[N][N]; // 起始状态
private static int[][] dist = new int[MAX_STATE][N]; // 到达每个状态的步数
private static int[][] pre = new int[MAX_STATE][N]; // 记录每个状态的前驱状态
private static int[][] vis = new int[MAX_STATE][N]; // 标记每个状态是否已经访问过
private static int[] pos = new int[9]; // 记录每个数字所在的位置
private static int getH(int[][] a) { // 计算估价函数h
int res = 0;
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (a[i][j] == 0) continue;
res += Math.abs(i - (a[i][j] - 1) / N) + Math.abs(j - (a[i][j] - 1) % N);
}
}
return res;
}
private static int getId(int[][] a) { // 将状态转化为整数
int res = 0;
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
res = res * 10 + a[i][j];
}
}
return res;
}
private static void printAns(int id) { // 输出解答
if (id == -1) return;
printAns(pre[id][0]);
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
System.out.print(start[i][j] + " ");
}
System.out.println();
}
System.out.println();
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
start[i][j] = sc.nextInt();
pos[start[i][j]] = i * N + j;
}
}
int startId = getId(start);
int goalId = getId(GOAL);
if ((getH(start) & 1) != (getH(GOAL) & 1)) { // 判断是否有解
System.out.println("No solution!");
return;
}
int hh = 0, tt = 0;
int[] q = new int[MAX_STATE];
Arrays.fill(dist, -1);
Arrays.fill(vis, 0);
dist[startId][0] = 0;
vis[startId][0] = 1;
q[0] = startId;
while (hh <= tt) {
int[][] cur = new int[N][N];
int t = q[hh++];
for (int i = N - 1; i >= 0; i--) {
for (int j = N - 1; j >= 0; j--) {
cur[i][j] = t % 10;
t /= 10;
}
}
if (getId(cur) == goalId) { // 到达目标状态
System.out.println("Steps: " + dist[goalId][0]);
printAns(goalId);
return;
}
int x = pos[0] / N, y = pos[0] % N;
for (int i = 0; i < 4; i++) { // 扩展状态
int nx = x + DIRS[i][0], ny = y + DIRS[i][1];
if (nx < 0 || nx >= N || ny < 0 || ny >= N) continue;
int[][] nxt = new int[N][N];
for (int j = 0; j < N; j++) {
for (int k = 0; k < N; k++) {
nxt[j][k] = cur[j][k];
}
}
nxt[x][y] = nxt[nx][ny];
nxt[nx][ny] = 0;
int nxtId = getId(nxt);
if (vis[nxtId][0] == 0) { // 新状态
vis[nxtId][0] = 1;
dist[nxtId][0] = dist[getId(cur)][0] + 1;
pre[nxtId][0] = getId(cur);
q[++tt] = nxtId;
}
}
}
System.out.println("No solution!"); // 无解
}
}
```
相关推荐
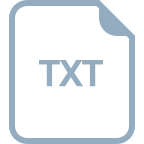
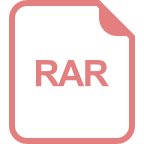







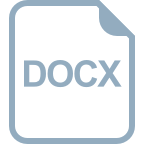