openwrt linux 多线程并发 特定线程挂起和释放 帮忙写一个C demo
时间: 2024-10-08 14:09:28 浏览: 29
OpenWRT Linux 是一个针对嵌入式设备定制的轻量级Linux发行版,它支持多线程并发处理。在 C 语言中,我们可以使用 pthreads 库来进行线程操作,包括创建、管理和同步。下面是一个简单的 C 示例,展示了如何创建两个线程,其中一个线程会被主动挂起并最终释放:
```c
#include <stdio.h>
#include <pthread.h>
// 线程函数
void* thread_function(void* arg) {
printf("Thread %d is running.\n", *(int*)arg);
// 模拟工作循环,这里我们简单地让线程休眠一段时间
sleep(5);
printf("Thread %d finished.\n", *(int*)arg);
return NULL;
}
int main() {
pthread_t thread1, thread2; // 线程ID
int id1 = 1, id2 = 2;
// 创建两个线程
if (pthread_create(&thread1, NULL, thread_function, &id1)) {
perror("Error creating thread1");
exit(EXIT_FAILURE);
}
if (pthread_create(&thread2, NULL, thread_function, &id2)) {
perror("Error creating thread2");
exit(EXIT_FAILURE);
}
// 主线程暂停一段时间
printf("Main thread pausing...\n");
sleep(3); // 挂起主线程
// 撤销挂起线程1
pthread_cancel(thread1);
// 等待线程结束(取消线程会立即返回)
pthread_join(thread1, NULL);
// 继续运行线程2
printf("Resuming normal operation and joining thread2...\n");
pthread_join(thread2, NULL);
printf("Both threads have completed.\n");
return 0;
}
```
在这个示例中,`main` 函数首先创建了两个线程,然后主线程短暂休息。此时,通过 `pthread_cancel` 取消线程1使其挂起,接着主线程继续执行。最后,主线程等待两个线程都完成。
阅读全文
相关推荐
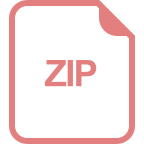
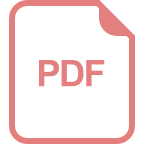
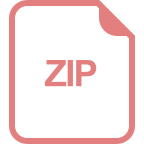


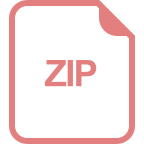
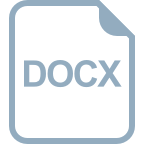
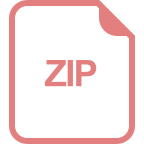
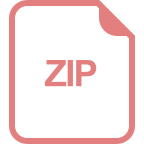
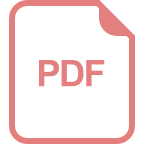
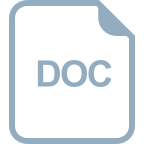