qt opengl 显示图片
时间: 2023-10-10 14:03:21 浏览: 93
Qt OpenGL可以用于显示图片,下面是一种常见的方法:
首先,需要在Qt程序中使用QOpenGLWidget类创建一个OpenGL的窗口。
```c++
#include <QApplication>
#include <QOpenGLWidget>
#include <QOpenGLFunctions>
#include <QOpenGLTexture>
#include <QImage>
#include <QPainter>
class OpenGLWidget : public QOpenGLWidget, protected QOpenGLFunctions
{
public:
OpenGLWidget(QWidget *parent = nullptr)
: QOpenGLWidget(parent)
{
}
protected:
void initializeGL() override
{
initializeOpenGLFunctions();
}
void paintGL() override
{
// 加载并绑定图片到OpenGL纹理对象
QOpenGLTexture *texture = new QOpenGLTexture(QImage("image.jpg"));
texture->bind();
// 使用纹理绘制图片
glBegin(GL_QUADS);
glTexCoord2f(0, 0); glVertex2i(-1, -1);
glTexCoord2f(1, 0); glVertex2i( 1, -1);
glTexCoord2f(1, 1); glVertex2i( 1, 1);
glTexCoord2f(0, 1); glVertex2i(-1, 1);
glEnd();
// 删除纹理对象
delete texture;
}
void resizeGL(int w, int h) override
{
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-1, 1, -1, 1, -1, 1);
glMatrixMode(GL_MODELVIEW);
}
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
OpenGLWidget openGLWidget;
openGLWidget.setWindowTitle("OpenGL显示图片");
openGLWidget.resize(800, 600);
openGLWidget.show();
return a.exec();
}
```
在这个例子中,我们使用QOpenGLTexture类来加载和绑定图片到OpenGL纹理对象,然后使用glTexCoord2f和glVertex2i函数在绘制图形时指定纹理坐标和顶点坐标,最后在绘制完成后删除纹理对象。
以上是一种常见的使用Qt OpenGL显示图片的方法,当然,还可以根据具体需求和场景进行相应的调整和扩展。
相关推荐
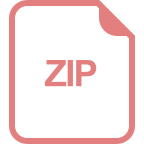














