帮我写一个个人电子笔记本系统代码,用vscold,需要前端代码要有日期标签,后端代码且用的是java代码,使用jsp框架,数据库代码。画出数据库E-R图,要有入录储存,日期检索功能
时间: 2024-10-13 17:09:11 浏览: 37
创建一个个人电子笔记本系统涉及到多个组件,包括前端界面、后端逻辑和数据库设计。以下是一个简化版的概述:
**前端(HTML + JavaScript, 使用VSCode)**
```html
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<title>Personal Notebook</title>
</head>
<body>
<div id="noteList">
<!-- 日期标签下的笔记列表将会在这里动态渲染 -->
</div>
<input type="date" id="datePicker" placeholder="Select a date">
<button onclick="addNote()">Add Note</button>
<script src="app.js"></script>
</body>
</html>
```
```javascript
// app.js (假设使用React或Vue)
function addNote() {
const selectedDate = document.getElementById('datePicker').value;
// 后端API调用
fetch('/notes/add', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ date: selectedDate, content: ... }),
})
.then(response => response.json())
.then(data => console.log(data));
}
```
**后端(Java, JSP)**
```java
// Controller (假设使用Spring Boot)
@Controller
public class NoteController {
@PostMapping("/notes/add")
public String addNote(@RequestParam String date, @RequestBody String content) {
// 这里处理数据库操作,添加笔记到对应日期的集合
saveToDatabase(date, content);
return "redirect:/";
}
private void saveToDatabase(String date, String content) {
// Java Persistence API (JPA) 或 Hibernate 操作
NotesRepository repository = new NotesRepository();
repository.save(new Note(date, content));
}
}
// JSP视图 (index.jsp)
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<c:forEach var="note" items="${notesByDate}">
<p>Date: ${note.date} - Content: ${note.content}</p>
</c:forEach>
```
**数据库设计(ER图)**
- 创建两个实体:`Note`(包含日期date和内容content字段)和`DateNotes`(关联表,存储每个日期的笔记)
- E-R图示例:

**数据库代码(SQL)**
```sql
CREATE TABLE Notes (
id INT PRIMARY KEY,
content TEXT NOT NULL,
date DATE NOT NULL
);
CREATE TABLE DateNotes (
date DATE PRIMARY KEY,
note_id INT,
FOREIGN KEY (note_id) REFERENCES Notes(id)
);
```
**日期检索功能**
在后端控制器中添加一个GET请求处理日期检索:
```java
@GetMapping("/notes/{date}")
public List<Note> getNotesByDate(@PathVariable String date) {
return notesRepository.findByDate(date);
}
```
在JSP视图中展示这些笔记:
```jsp
<c:forEach var="note" items="${notesByDate}">
...
</c:forEach>
```
**相关问题--:**
1. 如何在Spring Boot中集成数据库并设置JPA?
2. 前端如何实现与后端的交互,尤其是异步请求?
3. ER图中的关系如何映射到实际的数据库操作?
阅读全文
相关推荐
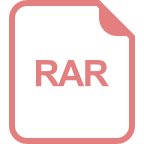
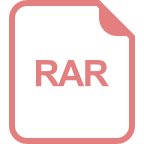
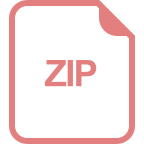





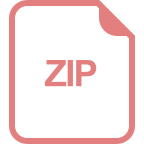
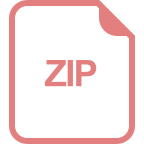
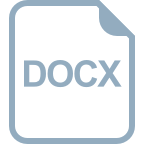
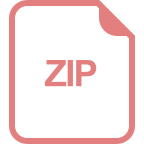
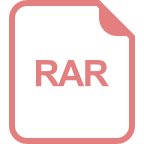
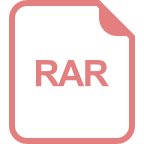
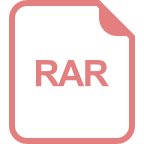
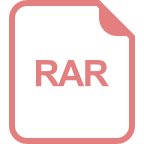