jar包读取外部配置文件
时间: 2024-09-09 17:12:54 浏览: 23
在Java中,当你需要从一个jar包中读取外部配置文件时,你可以采取以下步骤:
1. 确定配置文件的位置:首先,你需要确定配置文件的存放位置。配置文件通常放在项目的资源目录下(例如`src/main/resources`),打包成jar后,这些资源文件会位于jar包的`META-INF`目录下。
2. 获取资源流:在你的Java代码中,你可以使用类加载器(ClassLoader)来获取资源文件的输入流。可以使用`Thread.currentThread().getContextClassLoader()`或者`getClass().getClassLoader()`来获取当前线程的上下文类加载器或当前类的类加载器。
3. 读取文件内容:有了输入流之后,你可以使用IO流(如`BufferedReader`)来读取配置文件的内容。
下面是一个示例代码,展示如何在Java中读取位于`META-INF`目录下的配置文件`config.properties`:
```java
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.BufferedReader;
import java.util.Properties;
public class ConfigReader {
public static Properties readConfig() {
Properties config = new Properties();
try {
// 使用当前类的类加载器获取资源输入流
InputStream inputStream = ConfigReader.class.getClassLoader().getResourceAsStream("META-INF/config.properties");
if (inputStream == null) {
throw new RuntimeException("No config.properties found in the classpath.");
}
config.load(new InputStreamReader(inputStream, "UTF-8"));
} catch (Exception e) {
e.printStackTrace();
}
return config;
}
public static void main(String[] args) {
Properties config = readConfig();
// 输出读取到的配置信息,例如:
System.out.println(config.getProperty("key"));
}
}
```
请注意,配置文件读取可能会出现文件不存在、文件读取错误等情况,因此应当妥善处理这些异常情况。
相关推荐
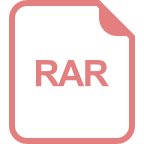
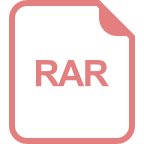
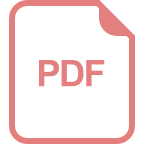














