nestjs rabbitmq 使用
时间: 2024-09-13 11:01:29 浏览: 54
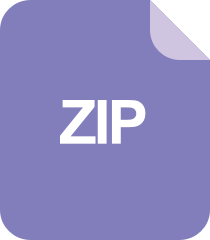
nestjs-rabbitmq
NestJS 是一个用于构建高效、可靠和可扩展的服务器端应用程序的框架。它使用 TypeScript 编写,但也兼容纯 JavaScript。NestJS 提供了对 RabbitMQ 的支持,RabbitMQ 是一个流行的开源消息代理软件,它实现了高级消息队列协议(AMQP)。
在 NestJS 中使用 RabbitMQ 通常会用到第三方库,如 `nestjs-typeorm-rabbitmq` 或者 `amqplib`,这里我们以 `amqplib` 为例说明如何集成 RabbitMQ 到 NestJS 应用中。
1. **安装依赖**:首先需要安装 `amqplib`,可以通过 npm 或 yarn 进行安装。
```bash
npm install amqplib
```
2. **创建 RabbitMQ 服务**:创建一个服务来封装与 RabbitMQ 的交互逻辑。
```typescript
// rabbitmq.service.ts
import * as amqp from 'amqplib';
export class RabbitMQService {
private connection: amqp.Connection;
private channel: amqp.Channel;
async connect(): Promise<void> {
this.connection = await amqp.connect('amqp://localhost');
this.channel = await this.connection.createChannel();
}
async publish(exchange: string, routingKey: string, content: string): Promise<void> {
await this.channel.assertExchange(exchange, 'direct', { durable: false });
this.channel.publish(exchange, routingKey, Buffer.from(content));
}
async close(): Promise<void> {
await this.channel.close();
await this.connection.close();
}
}
```
3. **使用服务**:在需要使用 RabbitMQ 的模块或控制器中注入这个服务,并调用相应的方法。
```typescript
// app.module.ts
import { Module } from '@nestjs/common';
import { RabbitMQService } from './rabbitmq.service';
import { AppController } from './app.controller';
@Module({
imports: [],
controllers: [AppController],
providers: [RabbitMQService],
})
export class AppModule {}
// app.controller.ts
import { Controller, Post } from '@nestjs/common';
import { RabbitMQService } from './rabbitmq.service';
@Controller()
export class AppController {
constructor(private rabbitMQService: RabbitMQService) {}
@Post('send')
async sendMessageToQueue() {
await this.rabbitMQService.connect();
await this.rabbitMQService.publish('exchangeName', 'routingKey', 'Hello, RabbitMQ!');
await this.rabbitMQService.close();
}
}
```
4. **配置和管理连接**:在实际应用中,连接的打开和关闭应该更加谨慎地管理,可能会使用到 NestJS 的生命周期钩子,如 `onModuleInit` 和 `onModuleDestroy`,来管理 RabbitMQ 连接的生命周期。
阅读全文
相关推荐
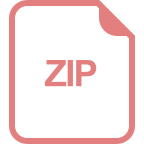
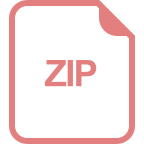


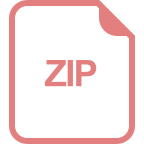
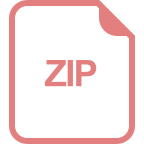
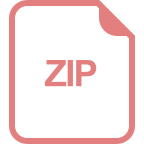
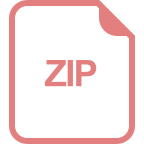
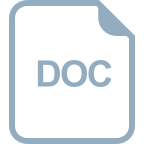
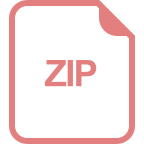
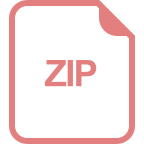
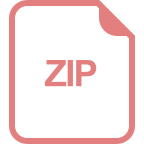
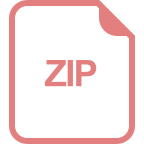
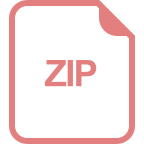
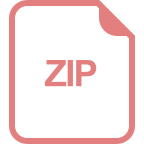
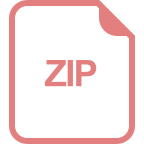
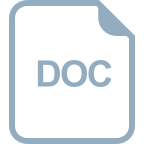
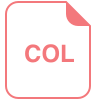