Connect to the USB stack (usbd_connect()) and provide two callbacks: one for insertion and one for removal. In the insertion callback: Connect to the USB device (usbd_attach()). Get descriptors (usbd_descriptor()). Select the configuration (usbd_select_config()) and interface (usbd_select_interface()). Set up communications pipes to the appropriate endpoint (usbd_open_pipe()). In the removal callback, detach from the USB device (usbd_detach()). Set up all data communications (e.g. reading and writing data, sending and receiving control information, etc.) via the usbd_setup_*() functions (usbd_setup_bulk(), usbd_setup_interrupt(), etc.). Initiate data transfer using the usbd_io() function (with completion callbacks if required). 这是官方的示例流程
时间: 2024-04-15 10:30:14 浏览: 245
感谢提供官方示例流程。根据你提供的流程,以下是使用 QNX 的 USB 库进行 USB 读卡器数据访问的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/iofunc.h>
#include <devctl.h>
#include <hw/usbdi.h>
int insertion_callback(usbd_device_t *dev, usbd_device_handle_t dev_handle, int iface_idx, int alt_idx)
{
// 连接到 USB 设备
if (usbd_attach(dev_handle, iface_idx, alt_idx) != EOK) {
fprintf(stderr, "Failed to attach USB device\n");
return -1;
}
// 获取设备描述符
if (usbd_descriptor(dev_handle) != EOK) {
fprintf(stderr, "Failed to get USB device descriptors\n");
usbd_detach(dev_handle);
return -1;
}
// 选择配置和接口
if (usbd_select_config(dev_handle, 0) != EOK) {
fprintf(stderr, "Failed to select USB device configuration\n");
usbd_detach(dev_handle);
return -1;
}
if (usbd_select_interface(dev_handle, iface_idx) != EOK) {
fprintf(stderr, "Failed to select USB device interface\n");
usbd_detach(dev_handle);
return -1;
}
// 设置通信管道
unsigned int ep_num = 1; // 假设使用端点1进行数据传输
usbd_pipe_handle_t pipe = usbd_open_pipe(dev_handle, ep_num);
if (pipe == NULL) {
fprintf(stderr, "Failed to open USB pipe\n");
usbd_detach(dev_handle);
return -1;
}
// 在此处可以进行数据传输等操作
return 0;
}
void removal_callback(usbd_device_t *dev, usbd_device_handle_t dev_handle)
{
// 从 USB 设备中断开连接
usbd_detach(dev_handle);
}
int main()
{
// 连接到 USB 栈
if (usbd_connect() != EOK) {
fprintf(stderr, "Failed to connect to USB stack\n");
return -1;
}
// 注册插入和移除回调函数
if (usbd_register_device_insertion_callback(insertion_callback) != EOK) {
fprintf(stderr, "Failed to register insertion callback\n");
return -1;
}
if (usbd_register_device_removal_callback(removal_callback) != EOK) {
fprintf(stderr, "Failed to register removal callback\n");
return -1;
}
// 在此处可以进行其他操作
// 等待 USB 设备插入和移除事件
while (1) {
sleep(1);
}
// 断开与 USB 栈的连接
usbd_disconnect();
return 0;
}
```
请注意,以上代码仅为示例,实际应用中可能需要根据具体的 USB 读卡器设备和要求进行相应的修改和调整。同时,还需要根据实际需求添加数据传输、读写等相关操作。建议参考 QNX 官方文档和示例代码以获取更详细和准确的信息。
阅读全文
相关推荐
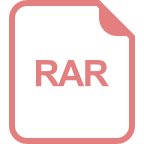
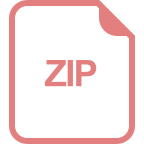
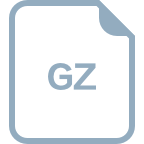
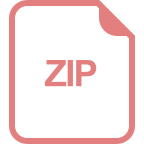
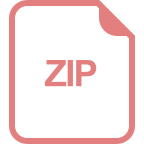
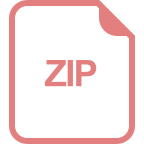
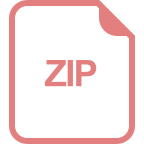
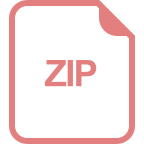
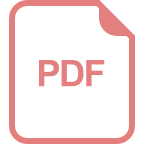
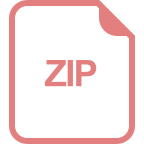
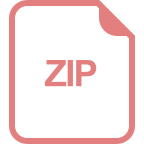
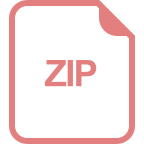
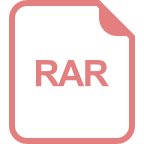
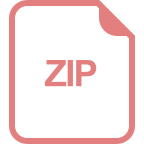
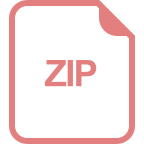
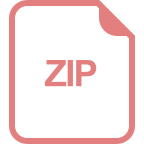