能否提供关于小猿口算的具体脚本代码或相关技术细节?
时间: 2024-10-24 21:13:27 浏览: 43
"小猿口算"通常是指一款教育软件或应用,它可能涉及到一些算法、图形用户界面(GUI)设计、数据结构(比如用于存储题目和答案的数据结构)、以及可能的在线评测功能等技术。为了编写这样的应用程序,我们需要使用到C++的一些关键部分:
1. **Qt** 或其他GUI库:用于构建交互式的窗口和界面元素,包括显示题目、接收用户输入和显示结果。
```cpp
#include <QApplication> // Qt基础库的一部分
#include <QLabel>
// 创建窗口并添加显示口算题目的标签
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QLabel *questionLabel = new QLabel("计算 5 + 7", &app);
// ...更多界面设置和布局代码
return app.exec();
}
```
2. **线程**:如果要实现实时的计算反馈,可能需要在后台线程进行口算,避免阻塞主线程。
```cpp
QThread *thread = new QThread;
Calculator worker; // 假设Calculator是处理计算的任务类
worker.moveToThread(thread); // 将计算任务移动到新线程
connect(thread, &QThread::started, &worker, &Calculator::startCalculation);
connect(&worker, &Calculator::resultReady, this, &MainWindow::displayResult);
thread->start(); // 开始计算
```
3. **数据结构**:用数组、列表或者队列来存储题目和答案,确保高效操作。
```cpp
std::vector<int> problems; // 存储口算题目
std::vector<int> answers; // 存储正确答案
// 添加题目
problems.push_back(5);
problems.push_back(7);
// 计算结果
int result = worker.calculate(problems.back());
answers.push_back(result);
```
4. **错误处理和评估**:检查用户输入是否有效,根据算法规则评分。
```cpp
bool isValidInput(const QString &input) {
// 检查输入是否为数字字符串并转换为整数
}
int evaluateAnswer(int userAnswer, int correctAnswer) {
if (isValidInput(userAnswer)) {
return (userAnswer == correctAnswer) ? score : wrongAnswerScore;
} else {
return invalidInputScore;
}
}
```
阅读全文
相关推荐
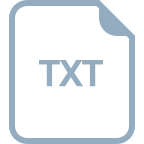
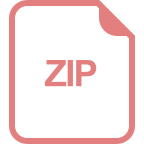





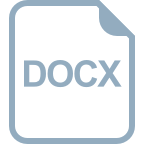









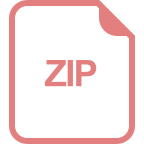