fastjson对象转字符串时候,忽略对象的某个属性,不使用注解实现
时间: 2024-10-21 10:11:25 浏览: 25
在使用Fastjson将对象转换为JSON字符串时,如果想要跳过对象的某个属性而不直接使用注解,你可以通过自定义`ObjectMapper`并实现`toJSONString`方法来达到目的。这里提供一种简单的做法:
首先,创建一个自定义的`JsonSerializer<T>`,针对需要忽略的属性重写序列化方法:
```java
import com.alibaba.fastjson.serializer.SerializerFeature;
import com.alibaba.fastjson.serializer.SimpleDateFormatDeserializer;
public class CustomJSONSerializer<T> extends JsonSerializer<T> {
private String ignoreProperty; // 要忽略的属性名
public CustomJSONSerializer(String ignoreProperty) {
this.ignoreProperty = ignoreProperty;
}
@Override
public void write(JSONWriter writer, T object, Object fieldName) {
if (object == null || !object.getClass().getDeclaredField(ignoreProperty).isAccessible()) return; // 检查字段是否可见
try {
Field field = object.getClass().getDeclaredField(ignoreProperty);
field.setAccessible(true); // 设置字段访问权限
field.set(object, null); // 将值设为null,使其在序列化时不包含
super.write(writer, object, fieldName);
} catch (IllegalAccessException e) {
throw new RuntimeException("Failed to set " + ignoreProperty + " to null", e);
}
}
}
```
然后,在实际序列化时,使用这个自定义的序列化器:
```java
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
public JSONObject toJSONWithoutProperty(Object obj, String ignoreProperty) {
CustomJSONSerializer<T> serializer = new CustomJSONSerializer<>(ignoreProperty);
return JSON.toJSONString(obj, new SerializerFeature.WriteDateUseDateFormat(), serializer);
}
// 使用示例
JSONObject jsonObject = toJSONWithoutProperty(yourObject, "yourIgnoredProperty");
```
这样,当你调用`toJSONString`时,指定的属性会被忽略。
阅读全文
相关推荐
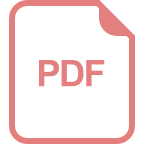
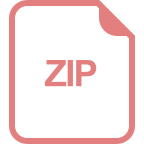
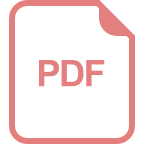
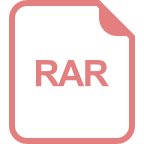
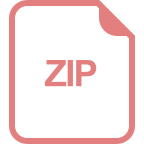
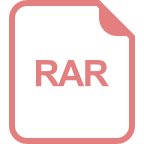
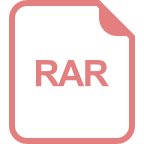
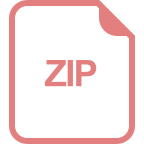
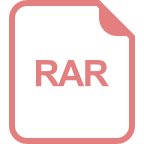
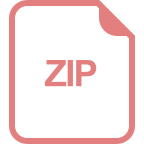
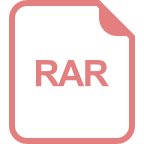
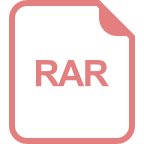
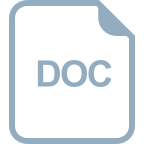
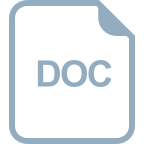
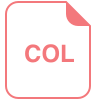




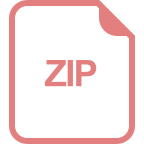