C++获取所有monitor名字,并修改第二个monitor缩放
时间: 2024-10-12 07:05:09 浏览: 26
在C++中,获取所有显示器名称并修改特定显示器的缩放比例通常涉及到操作系统级别的交互,这需要使用到Windows API或者跨平台的库(如Qt、Poco等)。这里是一个简化的示例,假设我们使用Windows API:
```cpp
#include <windows.h>
#include <iostream>
// 获取显示器列表函数
std::vector<std::string> getMonitorNames() {
DISPLAY_DEVICE devices[256];
DWORD count = sizeof(devices) / sizeof(DISPLAY_DEVICE);
if (EnumDisplayDevices(nullptr, 0, devices, count)) {
std::vector<std::string> monitorNames;
for (int i = 0; i < count; ++i) {
HMONITOR hMonitor = monitors[i].hMonitor;
MONITORINFO monitorInfo;
monitorInfo.cbSize = sizeof(MONITORINFO);
GetMonitorInfo(hMonitor, &monitorInfo);
monitorNames.push_back(monitorInfo.szDevice);
}
return monitorNames;
} else {
// 处理错误
return {};
}
}
// 修改显示器缩放函数,这里只是一个示例,实际操作可能更复杂
bool setMonitorScaling(const std::string& monitorName, int scalingFactor) {
HMONITOR hMonitor = MonitorFromLogicalWindow(HWND_DESKTOP, NULL); // 获取桌面的HMONITOR
if (!hMonitor) {
std::cerr << "Failed to find monitor by name: " << monitorName << std::endl;
return false;
}
MONITORINFOEX monitorInfo;
monitorInfo.cbSize = sizeof(MONITORINFOEX);
monitorInfo.rcMonitor.left = monitorInfo.rcMonitor.top = 0;
monitorInfo.rcMonitor.right = monitorInfo.rcMonitor.bottom = 0;
monitorInfo.dwFlags = MONITORINFOF_USE_DEFAULT_SIZE;
if (GetMonitorInfoEx(hMonitor, &monitorInfo)) { // 获取当前信息
monitorInfo.rcMonitor.right += scalingFactor * monitorInfo.rcMonitor.width / 100; // 修改宽度
monitorInfo.rcMonitor.bottom += scalingFactor * monitorInfo.rcMonitor.height / 100; // 修改高度
if (SetMonitorInfoEx(hMonitor, &monitorInfo, TRUE)) { // 设置新的信息
return true;
} else {
std::cerr << "Failed to change monitor scaling for " << monitorName << std::endl;
}
} else {
return false;
}
}
int main() {
std::vector<std::string> monitorNames = getMonitorNames();
if (!monitorNames.empty()) {
std::cout << "Available monitors:" << std::endl;
for (const auto& name : monitorNames) {
std::cout << name << std::endl;
}
// 修改第二个显示器的缩放比例
const int targetScaling = 120; // 比例调整百分比
if (setMonitorScaling(monitorNames[1], targetScaling)) {
std::cout << "Successfully changed the scaling of monitor " << monitorNames[1] << " to " << targetScaling << "%." << std::endl;
} else {
std::cout << "Failed to modify the monitor scaling." << std::endl;
}
}
return 0;
}
```
请注意,这个示例仅适用于Windows环境,且实际操作可能会受到权限限制,不是所有应用都能更改显示设置。
阅读全文
相关推荐
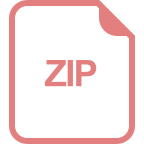
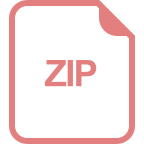
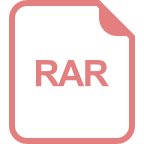

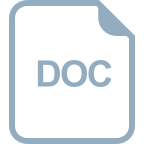
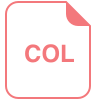



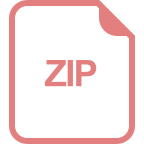
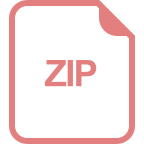
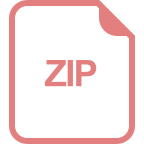
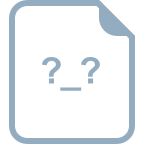
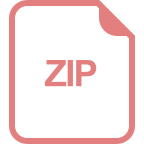