如何在C++中使用双线性插值算法实现BMP位图的无损缩放?请提供实现该功能的代码片段和步骤。
时间: 2024-10-31 22:26:32 浏览: 33
在处理BMP位图时,实现无损缩放是一项挑战,特别是在需要保持图像质量的同时改变其尺寸。为了解决这一问题,可以利用《C++实现BMP位图无损缩放代码分享》所提供的高效代码。这段代码将帮助你通过双线性插值算法来进行高质量的位图缩放。
参考资源链接:[C++实现BMP位图无损缩放代码分享](https://wenku.csdn.net/doc/6412b4a8be7fbd1778d405b5?spm=1055.2569.3001.10343)
双线性插值算法是一种广泛应用于图像处理中的插值技术,它通过利用最近的2x2像素的值来计算新像素的值,从而实现平滑的缩放效果。以下是实现该算法的关键步骤和代码片段:
1. 首先,你需要定义一个处理位图缩放的函数,比如`ScaleBMPBilinear`,它接受原始BMP图像的位图信息、目标宽度和高度。
```cpp
bool ScaleBMPBilinear(BITMAPINFOHEADER &bmihSrc, unsigned char *pBitsSrc, BITMAPINFOHEADER &bmihDst, unsigned char *pBitsDst, int newWidth, int newHeight)
{
// 源图像数据
int width = bmihSrc.biWidth;
int height = bmihSrc.biHeight;
// 目标图像数据
int dstWidth = newWidth;
int dstHeight = newHeight;
// 计算缩放比例
double xRatio = (double)width / dstWidth;
double yRatio = (double)height / dstHeight;
for (int y = 0; y < dstHeight; y++)
{
for (int x = 0; x < dstWidth; x++)
{
double xx = x * xRatio;
double yy = y * yRatio;
int x1 = (int)xx;
int y1 = (int)yy;
int x2 = min(x1 + 1, width - 1);
int y2 = min(y1 + 1, height - 1);
double xDiff = xx - x1;
double yDiff = yy - y1;
// 计算每个像素的R、G、B值
int red1 = pBitsSrc[(y1 * width + x1) * 3];
int green1 = pBitsSrc[(y1 * width + x1) * 3 + 1];
int blue1 = pBitsSrc[(y1 * width + x1) * 3 + 2];
int red2 = pBitsSrc[(y1 * width + x2) * 3];
int green2 = pBitsSrc[(y1 * width + x2) * 3 + 1];
int blue2 = pBitsSrc[(y1 * width + x2) * 3 + 2];
int red3 = pBitsSrc[(y2 * width + x1) * 3];
int green3 = pBitsSrc[(y2 * width + x1) * 3 + 1];
int blue3 = pBitsSrc[(y2 * width + x1) * 3 + 2];
int red4 = pBitsSrc[(y2 * width + x2) * 3];
int green4 = pBitsSrc[(y2 * width + x2) * 3 + 1];
int blue4 = pBitsSrc[(y2 * width + x2) * 3 + 2];
// 双线性插值计算新像素值
// ...
// 将新像素值写入目标位图
// ...
}
}
return true;
}
```
2. 在上述代码中,你需要填充双线性插值计算新像素值的部分。具体的计算方法涉及到红色、绿色和蓝色通道的插值,可以参考相关的图像处理文献来实现。
3. 最后,将计算出的像素值写入目标位图中。这涉及到正确地处理BMP位图的内存布局,确保颜色通道和像素对齐。
通过上述步骤,你可以在C++中使用双线性插值算法来实现BMP位图的无损缩放。这种方法在处理图像缩放时能够保持较高的图像质量,避免了传统像素复制方法所导致的失真和花点现象。为了进一步提高你的图像处理技能,建议深入学习图像处理相关的算法和优化技术。
参考资源链接:[C++实现BMP位图无损缩放代码分享](https://wenku.csdn.net/doc/6412b4a8be7fbd1778d405b5?spm=1055.2569.3001.10343)
阅读全文
相关推荐



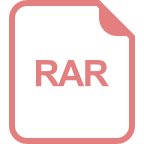

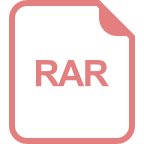
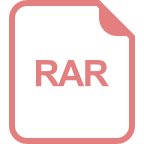
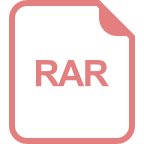


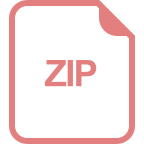






