c#控制台程序实现以下功能:读取一个文件,将文件内容读到一个string里面
时间: 2024-10-17 18:10:24 浏览: 24
在C#中,你可以使用`System.IO`命名空间下的`StreamReader`类来实现在控制台应用程序中读取文件并将其内容存储到字符串中。以下是简单的步骤:
1. 引入必要的命名空间:
```csharp
using System;
using System.IO;
```
2. 定义一个方法来读取文件:
```csharp
static string ReadFileToString(string filePath)
{
try
{
// 创建 StreamReader 对象来打开文件
using (StreamReader reader = new StreamReader(filePath))
{
// 逐行读取文件内容,并追加到字符串
StringBuilder contentBuilder = new StringBuilder();
string line;
while ((line = reader.ReadLine()) != null) // 当到达文件末尾时,readLine()会返回null
{
contentBuilder.AppendLine(line);
}
return contentBuilder.ToString(); // 返回整个文件的内容
}
}
catch (Exception ex)
{
Console.WriteLine($"Error reading file: {ex.Message}");
return null; // 如果发生错误,返回null表示失败
}
}
```
3. 调用该方法:
```csharp
string inputFile = "path_to_your_file.txt"; // 替换为你要读取的实际文件路径
string fileContent = ReadFileToString(inputFile);
if (fileContent != null)
{
Console.WriteLine("File content:");
Console.WriteLine(fileContent);
}
else
{
Console.WriteLine("Failed to read the file.");
}
```
在这个例子中,`ReadFileToString`函数会尝试打开指定的文件,如果成功则读取所有文本行并合并成一个字符串,然后返回给调用者。
阅读全文
相关推荐
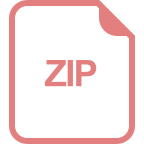
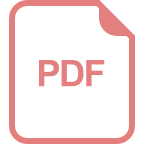
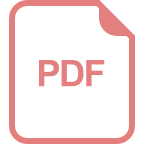
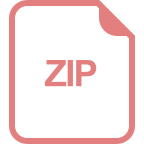
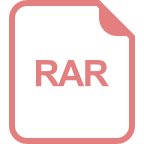
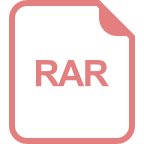
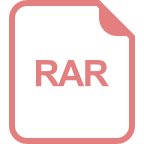
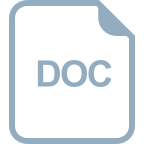
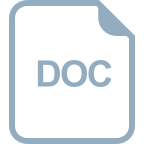
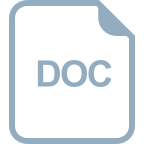
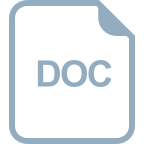







