c++ map insert重复
时间: 2023-11-03 13:57:15 浏览: 47
在C++的`std::map`容器中,当我们使用`insert`函数插入一个已经存在的键时,会发生什么取决于`map`的性质。`std::map`是一个关联容器,它按照键的顺序进行排序,并且每个键必须是唯一的。
如果使用`insert`函数插入一个已经存在的键,新的值将不会替换旧的值。相反,`insert`函数将返回一个`std::pair`对象,该对象的第一个成员指向已经存在的元素,并且第二个成员表示插入是否成功。
下面是一个示例代码,演示了这种情况:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap.insert(std::make_pair(1, "One"));
myMap.insert(std::make_pair(2, "Two"));
auto result = myMap.insert(std::make_pair(1, "New One"));
if (result.second) {
std::cout << "Insertion succeeded." << std::endl;
} else {
std::cout << "Insertion failed. Key already exists." << std::endl;
std::cout << "Existing value: " << result.first->second << std::endl;
}
return 0;
}
```
在上述代码中,我们首先插入了键值对`(1, "One")`和`(2, "Two")`。然后,我们再次尝试插入键值对`(1, "New One")`,但是由于键`1`已经存在,插入操作将失败。因此,我们在输出中显示了插入失败并打印了已存在的值。
输出结果为:
```
Insertion failed. Key already exists.
Existing value: One
```
希望这个例子能够帮助你理解当使用`insert`函数插入重复键时的行为。如果你还有其他问题,请随时提问。
相关推荐
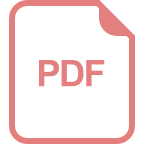
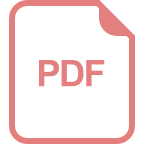
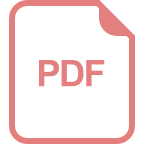














