springboot项目测试环境
时间: 2024-12-26 11:17:12 浏览: 2
### 配置和使用 Spring Boot 测试环境
#### 1. 默认依赖项引入
当创建一个新的 Spring Boot 项目时,默认情况下会包含 `spring-boot-starter-test` 这个依赖项,它主要用于支持单元测试功能。此依赖项包含了多个常用的库来帮助开发者编写高效的测试代码[^2]。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
```
上述 XML 片段展示了 Maven 构建工具中的 POM 文件里自动加入的部分内容。通过 `<scope>` 标签指定该依赖仅用于测试阶段,在生产环境中不会被打包进去。
#### 2. 编写简单的单元测试类
为了验证应用程序逻辑是否正常工作,可以利用 JUnit 来构建测试案例。下面是一个基于 Java 的简单例子:
```java
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class ExampleApplicationTests {
@Autowired
private MyService myService;
@Test
public void contextLoads() {
assertNotNull(myService);
}
}
```
这段代码展示了一个基本的集成测试方法,其中使用了 `@SpringBootTest` 注解加载整个应用上下文,并注入服务对象以便于后续操作。这里调用了 `assertNotNull()` 方法检查所获取的服务实例不是 null 值。
#### 3. 使用 MockMvc 进行 Web 层测试
对于涉及 HTTP 请求响应处理的功能模块,则可以通过模拟客户端请求的方式来进行更贴近实际场景下的测试。MockMvc 是一种非常方便的选择之一:
```java
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@Test
void shouldReturnDefaultMessage() throws Exception {
mockMvc.perform(get("/hello"))
.andExpect(status().isOk())
.andReturn();
}
```
在此处定义了一条 GET 类型 URL 路径 `/hello` 的访问行为预期返回状态码为 200 (OK),并执行相应的断言判断。
阅读全文
相关推荐
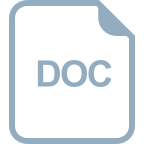
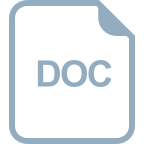
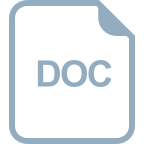
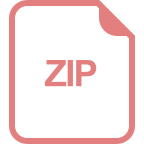
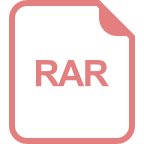
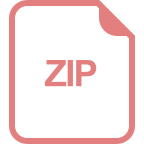
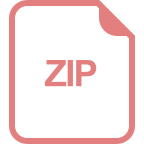
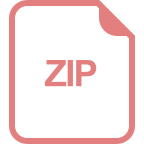
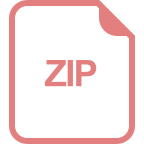
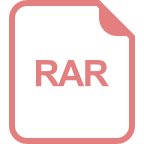
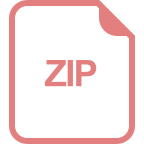
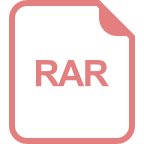
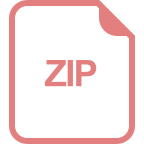
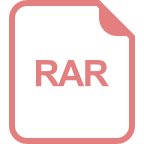
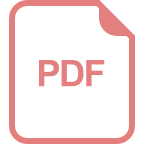
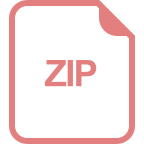
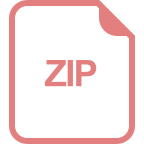
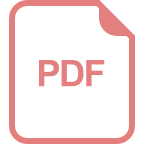
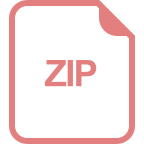