基于链表的图书信息管理,C语言源代码。
时间: 2024-12-29 12:32:12 浏览: 12
基于链表的图书信息管理通常会包含一个链表结构,用于存储书籍的数据,如书名、作者、出版社等。在C语言中,我们可以创建一个简单的图书节点结构,并提供相关的增删查改操作。以下是基础的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct BookNode {
char title[50];
char author[50];
char publisher[50];
struct BookNode* next;
} Book;
// 创建新节点函数
Book* createBookNode(char* title, char* author, char* publisher) {
Book* newNode = (Book*)malloc(sizeof(Book));
if (newNode != NULL) {
strcpy(newNode->title, title);
strcpy(newNode->author, author);
strcpy(newNode->publisher, publisher);
newNode->next = NULL;
}
return newNode;
}
// 添加书籍到链表
void addBook(Book** head, char* title, char* author, char* publisher) {
Book* newNode = createBookNode(title, author, publisher);
if (*head == NULL) {
*head = newNode;
} else {
Book* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表中的所有书籍
void printBooks(Book* head) {
Book* current = head;
while (current != NULL) {
printf("Title: %s, Author: %s, Publisher: %s\n", current->title, current->author, current->publisher);
current = current->next;
}
}
int main() {
Book* bookList = NULL;
// ... 使用上述函数添加书籍并打印
return 0;
}
阅读全文
相关推荐





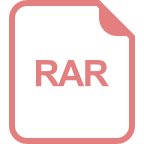
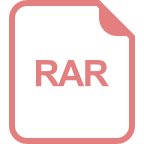

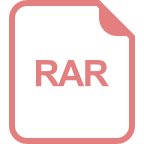

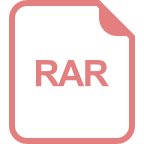



