html网页轮播banner
时间: 2023-12-09 12:35:43 浏览: 135
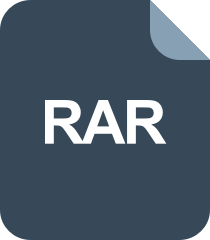
html网页轮播
以下是一个基于HTML5和CSS3的网页轮播banner的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML5 Banner</title>
<style>
/* 设置轮播图容器的宽度和高度 */
.banner {
width: 800px;
height: 400px;
position: relative;
margin: 0 auto;
overflow: hidden;
}
/* 设置轮播图中每张图片的样式 */
.banner img {
width: 100%;
height: 100%;
position: absolute;
top: 0;
left: 0;
opacity: 0;
transition: opacity 1s ease-in-out;
}
/* 设置轮播图中当前显示图片的样式 */
.banner img.active {
opacity: 1;
}
/* 设置轮播图中左右箭头的样式 */
.banner .arrow {
position: absolute;
top: 50%;
margin-top: -25px;
width: 50px;
height: 50px;
background-color: rgba(0, 0, 0, 0.5);
color: #fff;
text-align: center;
line-height: 50px;
font-size: 24px;
cursor: pointer;
transition: background-color 0.3s ease-in-out;
}
/* 设置轮播图中左右箭头的悬停样式 */
.banner .arrow:hover {
background-color: rgba(0, 0, 0, 0.8);
}
/* 设置轮播图中左箭头的样式 */
.banner .arrow.left {
left: 0;
}
/* 设置轮播图中右箭头的样式 */
.banner .arrow.right {
right: 0;
}
/* 设置轮播图中水纹效果的样式 */
.banner .ripple {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-image: radial-gradient(circle, #fff 10%, transparent 10.01%);
background-repeat: no-repeat;
background-position: 50%;
transform: scale(10, 10);
opacity: 0;
transition: transform 1s, opacity 1s;
}
/* 设置轮播图中当前显示图片的水纹效果的样式 */
.banner .ripple.active {
transform: scale(0, 0);
opacity: 0.3;
transition: none;
}
</style>
</head>
<body>
<div class="banner">
<img src="image1.jpg" alt="Image 1" class="active">
<img src="image2.jpg" alt="Image 2">
<img src="image3.jpg" alt="Image 3">
<div class="arrow left" onclick="prev()"><</div>
<div class="arrow right" onclick="next()">></div>
<div class="ripple"></div>
</div>
<script>
// 获取轮播图容器和所有图片元素
var banner = document.querySelector('.banner');
var images = banner.querySelectorAll('img');
// 获取左右箭头和水纹效果元素
var leftArrow = banner.querySelector('.arrow.left');
var rightArrow = banner.querySelector('.arrow.right');
var ripple = banner.querySelector('.ripple');
// 定义当前显示图片的索引
var currentIndex = 0;
// 定义自动播放的定时器
var timer = setInterval(next, 3000);
// 定义切换到下一张图片的函数
function next() {
// 获取当前显示图片和下一张图片的索引
var currentImage = images[currentIndex];
var nextIndex = (currentIndex + 1) % images.length;
var nextImage = images[nextIndex];
// 切换到下一张图片
currentImage.classList.remove('active');
nextImage.classList.add('active');
// 更新当前显示图片的索引
currentIndex = nextIndex;
// 显示水纹效果
showRipple();
}
// 定义切换到上一张图片的函数
function prev() {
// 获取当前显示图片和上一张图片的索引
var currentImage = images[currentIndex];
var prevIndex = (currentIndex - 1 + images.length) % images.length;
var prevImage = images[prevIndex];
// 切换到上一张图片
currentImage.classList.remove('active');
prevImage.classList.add('active');
// 更新当前显示图片的索引
currentIndex = prevIndex;
// 显示水纹效果
showRipple();
}
// 定义显示水纹效果的函数
function showRipple() {
// 获取当前显示图片的水纹效果元素
var currentRipple = ripple.cloneNode(true);
currentRipple.classList.add('active');
// 将水纹效果元素添加到轮播图容器中
banner.appendChild(currentRipple);
// 在下一帧中移除水纹效果元素
requestAnimationFrame(function() {
currentRipple.addEventListener('transitionend', function() {
currentRipple.remove();
});
currentRipple.style.transform = 'scale(1, 1)';
currentRipple.style.opacity = '0';
});
}
// 监听鼠标进入轮播图容器的事件,停止自动播放
banner.addEventListener('mouseenter', function() {
clearInterval(timer);
leftArrow.style.display = 'block';
rightArrow.style.display = 'block';
});
// 监听鼠标离开轮播图容器的事件,开始自动播放
banner.addEventListener('mouseleave', function() {
timer = setInterval(next, 3000);
leftArrow.style.display = 'none';
rightArrow.style.display = 'none';
});
</script>
</body>
</html>
```
阅读全文
相关推荐
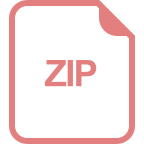
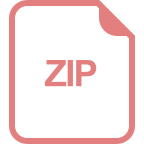
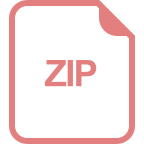
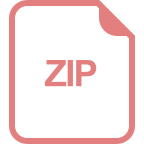
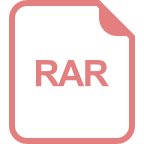
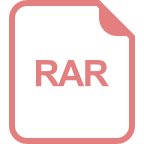
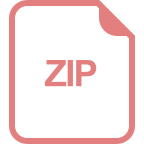
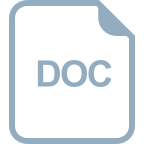
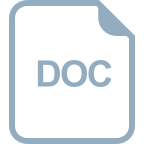

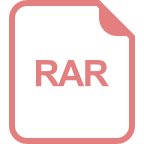
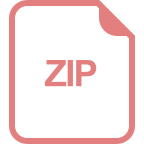
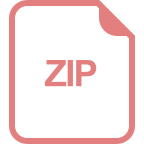
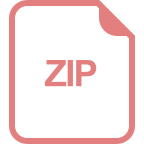
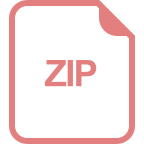
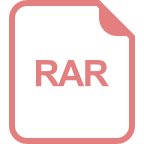