Python遗传算法解旅行商tsp问题
时间: 2024-05-28 20:07:49 浏览: 27
Python遗传算法可以用来解决旅行商问题(TSP),TSP问题是在一定数量的城市和它们之间的距离已知的情况下,求解访问每个城市一次且仅访问一次的最短路径。Python遗传算法可以通过以下步骤来解决该问题:
1. 定义基因编码:将每个城市编码成一个基因,并将每个基因随机组合成一个初始种群。
2. 定义适应度函数:计算每个个体的适应度值,即旅行商走过所有城市的距离之和。
3. 选择操作:选择适应度值高的个体,使其有更高的概率在下一代中出现。通常采用轮盘赌选择方法。
4. 交叉操作:将两个个体进行交叉,生成新的个体。可以采用单点交叉或多点交叉。
5. 变异操作:以一定的概率对新个体进行变异,增加种群的多样性。
6. 繁殖操作:根据选择、交叉和变异操作,生成下一代种群。
7. 终止条件:当达到最大迭代次数或者满足某种终止条件时,停止迭代并输出最优解。
相关问题:
1. 遗传算法是什么?
2. TSP问题是什么?有哪些解决方法?
3. 遗传算法如何实现TSP问题的求解?
4. 如何评价TSP问题的求解效果?
相关问题
python遗传算法旅行商tsp
好的,关于python遗传算法解决旅行商问题(TSP),你需要先了解遗传算法的基本原理和TSP问题的定义。遗传算法是一种基于自然选择和遗传机制的优化算法,而TSP问题则是指在给定的一组城市和它们之间的距离,找到一条最短的路径,使得每个城市恰好被访问一次。
在python中,你可以使用遗传算法库DEAP来实现TSP问题的求解。具体步骤如下:
1. 定义适应度函数:即计算每个个体(路径)的适应度值,这里可以使用路径长度作为适应度值。
2. 定义遗传算法的参数:包括种群大小、交叉概率、变异概率等。
3. 定义遗传算法的操作:包括选择、交叉和变异操作。
4. 运行遗传算法:通过迭代运行遗传算法,不断优化种群中的个体,直到达到预设的停止条件。
下面是一个简单的示例代码:
```python
import random
from deap import base, creator, tools
# 定义TSP问题
cities = [(0, 0), (1, 5), (2, 3), (5, 4), (6, 1)]
# 定义适应度函数
def evalTSP(individual):
distance = 0
for i in range(len(individual)):
j = (i + 1) % len(individual)
city_i = cities[individual[i]]
city_j = cities[individual[j]]
distance += ((city_i[0] - city_j[0]) ** 2 + (city_i[1] - city_j[1]) ** 2) ** 0.5
return distance,
# 定义遗传算法参数
POPULATION_SIZE = 100
P_CROSSOVER = 0.9
P_MUTATION = 0.1
MAX_GENERATIONS = 100
HALL_OF_FAME_SIZE = 10
# 创建遗传算法工具箱
toolbox = base.Toolbox()
# 创建个体和种群
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox.register("attr_int", random.sample, range(len(cities)), len(cities))
toolbox.register("individual", tools.initIterate, creator.Individual, toolbox.attr_int)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 注册遗传算法操作
toolbox.register("evaluate", evalTSP)
toolbox.register("mate", tools.cxOrdered)
toolbox.register("mutate", tools.mutShuffleIndexes, indpb=1.0/len(cities))
toolbox.register("select", tools.selTournament, tournsize=3)
# 运行遗传算法
population = toolbox.population(n=POPULATION_SIZE)
hof = tools.HallOfFame(HALL_OF_FAME_SIZE)
stats = tools.Statistics(lambda ind: ind.fitness.values)
stats.register("avg", tools.mean)
stats.register("min", tools.min)
algorithms.eaSimple(population, toolbox, cxpb=P_CROSSOVER, mutpb=P_MUTATION, ngen=MAX_GENERATIONS, stats=stats, halloffame=hof)
# 输出结果
best = hof[0]
print("Best individual is ", best)
print("with fitness ", best.fitness.values[0])
```
python实现遗传算法tsp旅行商问题
以下是Python实现遗传算法解决TSP旅行商问题的示例代码:
```python
import random
# 城市坐标
city_pos = [(60, 200), (180, 200), (80, 180), (140, 180), (20, 160), (100, 160), (200, 160), (140, 140), (40, 120), (100, 120), (180, 100), (60, 80), (120, 80), (180, 60), (20, 40), (100, 40), (200, 40), (20, 20), (60, 20), (160, 20)]
# 种群大小
POP_SIZE = 500
# 城市数量
ITY_COUNT = len(city_pos)
# 交叉概率
CROSS_RATE = 0.1
# 变异概率
MUTATION_RATE = 0.02
# 代数
N_GENERATIONS = 500
# 计算两个城市之间的距离
def distance(city1, city2):
return ((city1[0] - city2[0]) ** 2 + (city1[1] - city2[1]) ** 2) ** 0.5
# 计算一条路径的总距离
def get_fitness(path):
distance_sum = 0
for i in range(CITY_COUNT - 1):
distance_sum += distance(city_pos[path[i]], city_pos[path[i+1]])
distance_sum += distance(city_pos[path[-1]], city_pos[path[0]])
return 1 / distance_sum
# 初始化种群
def init_population():
population = []
for i in range(POP_SIZE):
path = list(range(CITY_COUNT))
random.shuffle(path)
population.append(path)
return population
# 选择
def select(population, fitness):
idx = random.randint(0, POP_SIZE - 1)
for i in range(POP_SIZE):
if random.random() < fitness[i] / fitness.sum():
idx = i
break
return population[idx]
# 交叉
def crossover(parent1, parent2):
if random.random() < CROSS_RATE:
child = [-1] * CITY_COUNT
start = random.randint(0, CITY_COUNT - 1)
end = random.randint(start, CITY_COUNT - 1)
child[start:end+1] = parent1[start:end+1]
for i in range(CITY_COUNT):
if parent2[i] not in child:
for j in range(CITY_COUNT):
if child[j] == -1:
child[j] = parent2[i]
break
return child
else:
return parent1
# 变异
def mutate(child):
if random.random() < MUTATION_RATE:
idx1, idx2 = random.sample(range(CITY_COUNT), 2)
child[idx1], child[idx2] = child[idx2], child[idx1]
return child
# 遗传算法主函数
def genetic_algorithm():
population = init_population()
for generation in range(N_GENERATIONS):
fitness = [get_fitness(path) for path in population]
best_path = population[fitness.index(max(fitness))]
print("Generation:", generation, "| Best path length:", 1 / max(fitness))
new_population = [best_path]
for i in range(POP_SIZE - 1):
parent1 = select(population, fitness)
parent2 = select(population, fitness)
child = crossover(parent1, parent2)
child = mutate(child)
new_population.append(child)
population = new_population
return best_path
# 运行遗传算法
best_path = genetic_algorithm()
print("Best path:", best_path)
```
相关推荐
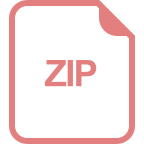












