react this.$router.go(-1);
时间: 2024-04-24 20:26:15 浏览: 16
在React中,我们使用react-router来进行路由管理。如果你想在React组件中实现类似于`this.$router.go(-1)`的功能,可以按照以下步骤操作:
1. 首先,确保你已经安装了`react-router-dom`依赖包。你可以使用以下命令进行安装:
```shell
npm install react-router-dom
```
2. 在你的组件文件中引入`BrowserRouter`和`useHistory`:
```javascript
import { BrowserRouter, useHistory } from "react-router-dom";
```
3. 在组件函数内部使用`useHistory`钩子来获取路由历史对象:
```javascript
const history = useHistory();
```
4. 然后,你可以通过调用`history.goBack()`方法来返回上一个路由页面:
```javascript
history.goBack();
```
这样就可以实现在React中返回上一个页面的功能了。希望对你有所帮助!
相关问题
react history.push 代码 demo
以下是一个简单的 React 应用程序中使用 `history.push()` 进行页面跳转的示例代码:
```jsx
import React from 'react';
import { BrowserRouter as Router, Switch, Route, Link, useHistory } from 'react-router-dom';
const Home = () => {
const history = useHistory();
const handleClick = () => {
history.push('/about');
};
return (
<div>
<h1>Home Page</h1>
<button onClick={handleClick}>Go to About Page</button>
</div>
);
};
const About = () => {
return (
<div>
<h1>About Page</h1>
<p>This is the about page.</p>
<Link to="/">Go back to Home Page</Link>
</div>
);
};
const App = () => {
return (
<Router>
<Switch>
<Route exact path="/">
<Home />
</Route>
<Route path="/about">
<About />
</Route>
</Switch>
</Router>
);
};
export default App;
```
在上面的示例代码中,我们首先导入 `useHistory` 钩子,然后在 `Home` 组件中使用 `useHistory` 钩子来获取 `history` 对象。我们定义了一个 `handleClick` 函数,在点击按钮时使用 `history.push('/about')` 来跳转到关于页面。在 `About` 组件中,我们使用 `Link` 组件来返回到主页。最后,我们将 `Home` 和 `About` 组件包装在 `Switch` 和 `Route` 组件中,并将其添加到 `Router` 组件中。
taro如何接入react-router
Taro 是一个类 React 的跨端框架,支持使用 React 的语法编写页面。如果要在 Taro 中接入 React Router,可以按照以下步骤操作:
1. 安装 `react-router-dom`:
```bash
npm install react-router-dom --save
```
2. 在 `app.js` 中引入 `BrowserRouter` 并包裹 `Provider` 和 `Router`:
```javascript
import Taro, { Component } from '@tarojs/taro'
import { Provider } from '@tarojs/redux'
import { BrowserRouter as Router } from 'react-router-dom'
import configStore from './store'
import './app.less'
const store = configStore()
class App extends Component {
componentDidMount () {}
componentDidShow () {}
componentDidHide () {}
componentDidCatchError () {}
render () {
return (
<Provider store={store}>
<Router>
{this.props.children}
</Router>
</Provider>
)
}
}
export default App
```
3. 在页面中使用 `Route`、`Link` 等组件:
```javascript
import Taro, { Component } from '@tarojs/taro'
import { View, Text } from '@tarojs/components'
import { Link, Route, Switch } from 'react-router-dom'
import './index.less'
class Index extends Component {
render () {
return (
<View className='index'>
<Text>Hello world!</Text>
<Link to='/detail'>Go to detail page</Link>
<Switch>
<Route path='/detail' component={Detail} />
</Switch>
</View>
)
}
}
class Detail extends Component {
render () {
return (
<View className='detail'>
<Text>Detail page</Text>
<Link to='/'>Go back to index page</Link>
</View>
)
}
}
export default Index
```
通过以上步骤,就可以在 Taro 中接入 React Router 了,实现更加灵活的路由控制。
相关推荐
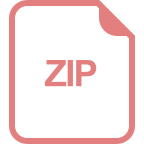
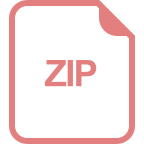
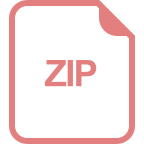












