我想用post请求,在spring boot中输入一串字符,应该如何写代码
时间: 2024-09-25 09:16:48 浏览: 12
在Spring Boot中,你可以使用`RestTemplate`或者`@RestController`的`PostMapping`注解来进行POST请求。下面是两个示例:
**使用`RestTemplate`(手动创建HTTP客户端):**
```java
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class MyService {
private final RestTemplate restTemplate = new RestTemplate();
public ResponseEntity<String> sendPostRequest(String input) {
String url = "http://your-api-url"; // 替换为你实际的API地址
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
HttpEntity<String> request = new HttpEntity<>(input, headers);
return restTemplate.postForEntity(url, request, String.class);
}
}
```
**使用`@RestController`(基于Spring MVC):**
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate; // 可能需要导入这个依赖
@RestController
public class MyController {
@Autowired
private RestTemplate restTemplate;
@PostMapping("/api endpoint")
public ResponseEntity<String> postRequest(@RequestBody String input) {
String url = "http://your-api-url"; // 同上
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
return restTemplate.exchange(url, HttpMethod.POST, new HttpEntity<>(input, headers), String.class);
}
}
```
在这两个例子中,你需要将URL替换为你实际的后端服务接收POST请求的地址,并确保服务器配置支持JSON内容类型。
相关推荐
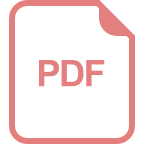
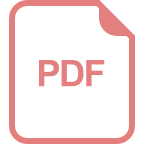
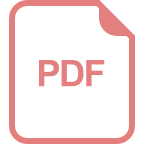














