代码实现QT ctrl+F添加
时间: 2024-12-15 17:28:06 浏览: 6
在Qt中,Ctrl+F通常是用于搜索文本的功能,通常在文本编辑器或类似组件中实现。如果你想在Qt应用程序中提供类似功能,你可以使用QShortcut和QLineEdit结合。以下是一个简单的步骤来创建这个功能:
1. 首先,在`.ui`文件中,如果你有一个QLineEdit控件,确保它已经被关联到相应的槽函数。
2. 在你的`.cpp`文件中,包括必要的头文件:
```cpp
#include <QShortcut>
#include <QLineEdit>
```
3. 在`QObject`的派生类中设置快捷键和对应的槽函数,例如:
```cpp
QShortcut *findShortcut = new QShortcut(QKeySequence("Ctrl+F"), this);
connect(findShortcut, &QShortcut::activated, yourLineEdit, &QLineEdit::selectAll); // 选择所有文本
```
这里,`yourLineEdit`是你的QLineEdit实例名。
4. 当用户按下Ctrl+F组合键时,`selectAll()`方法会被调用,这将选中文本框内的全部内容。
5. 如果你想实现全文搜索,你需要额外的逻辑来处理搜索请求,这可能涉及到遍历整个文档或其他数据源。
相关问题
pyside6 中怎么通过Ctrl+F弹出搜索框
在PySide6中,可以通过QShortcut类捕获键盘快捷键事件,然后在事件处理函数中执行相应的操作。具体实现方法如下:
```python
from PySide6.QtCore import Qt, QShortcut
from PySide6.QtWidgets import QApplication, QLineEdit, QMainWindow
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 创建搜索框
self.search_box = QLineEdit(self)
self.setCentralWidget(self.search_box)
# 创建Ctrl+F快捷键
shortcut = QShortcut(Qt.CTRL + Qt.Key_F, self)
shortcut.activated.connect(self.show_search_box)
def show_search_box(self):
# 在此处添加弹出搜索框的代码
print("Ctrl+F pressed")
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec()
```
在上面的代码中,我们首先创建了一个QLineEdit对象作为主窗口的中心部件,并将其设置为窗口的中心部件。然后,我们创建了一个QShortcut对象,将其绑定到Ctrl+F组合键上,并将其连接到show_search_box()方法上。当用户按下Ctrl+F组合键时,QShortcut对象会发出activated信号,触发show_search_box()方法。
在show_search_box()方法中,我们可以添加弹出搜索框的代码。在这个例子中,我们只是简单地打印一条消息来模拟弹出搜索框的效果。你可以根据自己的需求来实现弹出搜索框的具体逻辑。
python+qt实现记事本
以下是使用Python和Qt实现记事本的基本步骤:
1.安装PyQt库
PyQt是一个Python库,用于创建GUI应用程序。它可以在PyPI上通过pip包管理器安装。
2.创建一个Qt窗口应用程序
使用Qt Designer创建一个窗口,添加文本编辑器和菜单栏等必要的组件。
3.在Python中加载Qt窗口
使用PyQt加载Qt窗口,将窗口上的组件与Python代码连接。
4.添加文件读写功能
为了让用户能够打开和保存文件,需要添加文件读写功能。
5.添加其他功能
根据需求,可以添加其他功能,如剪切、复制和粘贴等。
以下是Python代码的示例:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QAction, QFileDialog
class Notepad(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.textEdit = QTextEdit()
self.setCentralWidget(self.textEdit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu('File')
openFile = QAction('Open', self)
openFile.setShortcut('Ctrl+O')
openFile.triggered.connect(self.showDialog)
saveFile = QAction('Save', self)
saveFile.setShortcut('Ctrl+S')
saveFile.triggered.connect(self.saveDialog)
fileMenu.addAction(openFile)
fileMenu.addAction(saveFile)
self.setGeometry(300, 300, 350, 300)
self.setWindowTitle('Notepad')
self.show()
def showDialog(self):
fname = QFileDialog.getOpenFileName(self, 'Open file', '/home')
if fname[0]:
f = open(fname[0], 'r')
with f:
data = f.read()
self.textEdit.setText(data)
def saveDialog(self):
fname = QFileDialog.getSaveFileName(self, 'Save file', '/home')
if fname[0]:
f = open(fname[0], 'w')
with f:
f.write(self.textEdit.toPlainText())
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Notepad()
sys.exit(app.exec_())
阅读全文
相关推荐
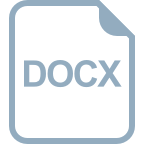
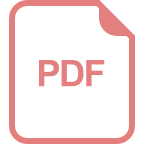
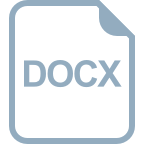













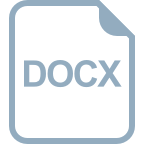