c#socket编程ftp
时间: 2023-08-09 08:08:18 浏览: 178
您好!对于C#中的Socket编程来实现FTP功能,您可以使用System.Net命名空间中的FtpWebRequest和FtpWebResponse类。下面是一个简单的示例代码,演示了如何使用Socket编程实现FTP上传和下载文件的功能:
```csharp
using System;
using System.IO;
using System.Net;
class FTPExample
{
static void Main()
{
// FTP服务器地址
string ftpServer = "ftp://ftp.example.com/";
// FTP登录用户名
string ftpUsername = "username";
// FTP登录密码
string ftpPassword = "password";
// 上传文件到FTP服务器
UploadFile(ftpServer, ftpUsername, ftpPassword, "localfile.txt", "remotefile.txt");
// 下载文件从FTP服务器
DownloadFile(ftpServer, ftpUsername, ftpPassword, "remotefile.txt", "localfile.txt");
Console.WriteLine("完成!");
}
static void UploadFile(string ftpServer, string ftpUsername, string ftpPassword, string localFilePath, string remoteFileName)
{
FtpWebRequest ftpRequest = (FtpWebRequest)WebRequest.Create(ftpServer + remoteFileName);
ftpRequest.Method = WebRequestMethods.Ftp.UploadFile;
ftpRequest.Credentials = new NetworkCredential(ftpUsername, ftpPassword);
using (FileStream localFileStream = new FileStream(localFilePath, FileMode.Open))
using (Stream ftpStream = ftpRequest.GetRequestStream())
{
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = localFileStream.Read(buffer, 0, buffer.Length)) > 0)
{
ftpStream.Write(buffer, 0, bytesRead);
}
}
}
static void DownloadFile(string ftpServer, string ftpUsername, string ftpPassword, string remoteFileName, string localFilePath)
{
FtpWebRequest ftpRequest = (FtpWebRequest)WebRequest.Create(ftpServer + remoteFileName);
ftpRequest.Method = WebRequestMethods.Ftp.DownloadFile;
ftpRequest.Credentials = new NetworkCredential(ftpUsername, ftpPassword);
using (FtpWebResponse ftpResponse = (FtpWebResponse)ftpRequest.GetResponse())
using (Stream ftpStream = ftpResponse.GetResponseStream())
using (FileStream localFileStream = new FileStream(localFilePath, FileMode.Create))
{
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = ftpStream.Read(buffer, 0, buffer.Length)) > 0)
{
localFileStream.Write(buffer, 0, bytesRead);
}
}
}
}
```
在上述代码中,`UploadFile`方法用于上传本地文件到FTP服务器,`DownloadFile`方法用于从FTP服务器下载文件到本地。您可以根据需要进行调整和扩展。
请注意,此示例使用了明文的用户名和密码,这在实际应用中是不安全的。建议您使用安全加密的方式(例如SFTP)来处理FTP连接和身份验证。
希望能对您有所帮助!如果您还有其他问题,请随时提问。
阅读全文
相关推荐
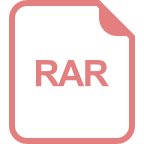
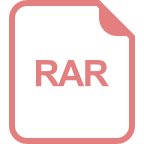

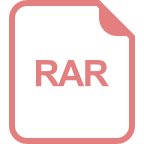
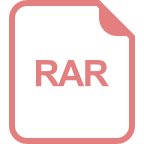
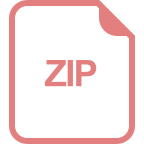

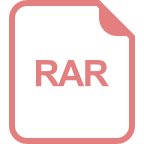
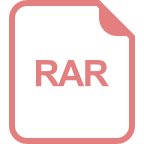







