用python 遍历创建名字为1,2,3,...,33 的文件夹,在文件夹中创建a.txt和b.txt文件,向a.txt文件第一行写入a,第二行写入b,第三行写入 c,向b.txt文件第一行写入a1,第二行写入b1,第三行写入c1
时间: 2024-04-14 13:26:54 浏览: 42
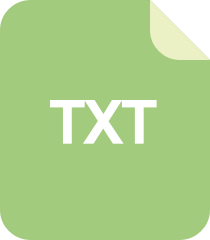
python遍历文件夹下文件,并生成txt
好的,我将为您提供一个Python代码示例来完成这个任务:
```python
import os
# 遍历创建文件夹
for i in range(1, 34):
folder_name = str(i)
os.makedirs(folder_name)
# 创建a.txt并写入内容
with open(os.path.join(folder_name, 'a.txt'), 'w') as file_a:
file_a.write('a\n')
file_a.write('b\n')
file_a.write('c\n')
# 创建b.txt并写入内容
with open(os.path.join(folder_name, 'b.txt'), 'w') as file_b:
file_b.write('a1\n')
file_b.write('b1\n')
file_b.write('c1\n')
```
这段代码使用 `os` 模块遍历创建名字为 1 到 33 的文件夹。在每个文件夹中,使用 `open` 函数创建 `a.txt` 和 `b.txt` 文件,并向文件中写入指定的内容。
请确保代码运行的目录具有足够的权限以创建文件夹和写入文件。
阅读全文
相关推荐







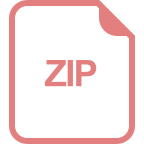
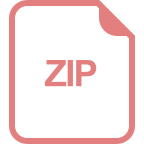
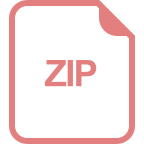
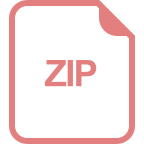
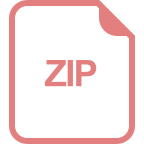