unity写一个方法,两个链表一起相减,直到一个链表为空
时间: 2024-10-27 22:06:00 浏览: 22
在Unity中,由于游戏引擎本身并不支持链表操作,链表通常用于数据结构的学习和算法实现,而不是直接在游戏中使用。不过,如果你需要模拟链表并在脚本中编写这样的功能,你可以创建自定义的数据结构和类来表示链表节点,并定义一个递归的方法来进行链表相减。
首先,你需要定义一个`ListNode`类,它包含一个值`value`和一个指向下一个节点的引用`next`:
```csharp
public class ListNode
{
public int value;
public ListNode next;
public ListNode(int value)
{
this.value = value;
this.next = null;
}
}
```
然后,可以创建一个`LinkedList`类,包含一个头节点`head`:
```csharp
public class LinkedList
{
public ListNode head;
// 添加节点的方法省略...
}
```
接下来,我们可以定义一个名为` SubtractLists`的方法,该方法接收两个链表作为输入,通过遍历并逐个比较节点的值来减去它们:
```csharp
public ListNode SubtractLists(LinkedList list1, LinkedList list2)
{
if (list1.head == null) return list2.head; // 如果list1为空,则返回list2
else if (list2.head == null) return list1.head; // 如果list2为空,则返回list1
ListNode resultHead = new ListNode(list1.head.value - list2.head.value); // 创建结果链表的头部
ListNode temp = resultHead;
ListNode node1 = list1.head.next;
ListNode node2 = list2.head.next;
while (node1 != null && node2 != null)
{
temp.next = new ListNode(node1.value - node2.value);
temp = temp.next;
node1 = node1.next;
node2 = node2.next;
}
// 将剩余的其中一个链表的节点添加到结果中,如果还有剩余
while (node1 != null || node2 != null)
{
if (node1 != null)
{
temp.next = node1;
node1 = node1.next;
}
else
{
temp.next = node2;
node2 = node2.next;
}
temp = temp.next;
}
return resultHead;
}
```
最后,记得处理特殊情况,如其中一个链表为空的情况。
阅读全文
相关推荐










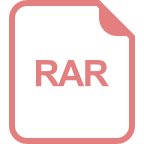




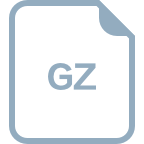