写出v-for指令的三种遍历方法所使用的语句。
时间: 2024-11-15 07:32:04 浏览: 15
在Vue.js中,`v-for`指令用于循环遍历数组或其他可迭代的数据源。主要有以下三种常用的遍历方法:
1. **基于索引的遍历**:
```html
<ul>
<li v-for="(item, index) in items" :key="index">
{{ item }}
</li>
</ul>
```
这里,`item`表示当前元素的值,`index`表示当前元素的索引。
2. **基于对象属性的遍历**:
```html
<div v-for="(user, userId) in users" :key="userId">
<p>{{ user.name }}</p>
</div>
```
在这种情况下,`user`是一个对象,我们可以直接访问其属性,如`name`。
3. **自定义迭代对象(对象映射)**:
```html
<template v-for="{ key, value } in objectMap" :key="key">
<div>{{ key }}: {{ value }}</div>
</template>
```
如果数据源是对象映射,你可以通过键值对的形式访问,`key`代表键名,`value`代表对应的值。
相关问题
使用c++语言写一个8位数据,每一位都代表不同的状态。写出一段代码,可以合理判定这8位数据处于什么状态,并给出相应的指令
在C++中,我们可以创建一个八位二进制数(unsigned char类型)来表示8位的状态。每个位都可以通过按位运算符来进行检查。下面是一个简单的代码示例,它会判断这个8位数据的状态并给出对应的指令:
```cpp
#include <iostream>
// 定义一个八位数据结构
unsigned char eightBitData;
// 状态定义
enum BitState {
LOW = 0, // 位值为0
HIGH = 1 // 位值为1
};
void checkAndDisplayStatus() {
for (int i = 0; i < 8; ++i) {
bool isHigh = (eightBitData & (1 << i)); // 检查第i位是否为1
if (isHigh) {
std::cout << "第" << i+1 << "位为高(1): ";
switch (i) { // 对于每一种状态,执行相应的指令
case 0:
std::cout << "指令A";
break;
case 1:
std::cout << "指令B";
break;
// 添加更多case for 其他位的指令...
default:
std::cout << "未知指令";
break;
}
std::cout << "\n";
} else {
std::cout << "第" << i+1 << "位为低(0)\n";
}
}
}
int main() {
// 初始化你的8位数据
eightBitData = 0b01010101; // 或者从用户输入获取
checkAndDisplayStatus();
return 0;
}
```
在这个例子中,我们首先定义了一个8位数据`eightBitData`,然后遍历每一个位。如果某一位为1(`isHigh`为真),则显示该位的索引以及对应的指令。请注意,你需要根据实际需求替换`switch`语句中的指令。
<template > <div class="product-wrap" > <div class="product" v-for="product in products" :key="product.id" @click="handleClick(product.id)"> <img :src="product.image" alt="" > <div> <span>{{ product.name }}</span> <h4>¥{{ product.price }}</h4> </div> <component :is="currentComponent"></component> </div> </div> </template> <script> export default { name: 'GoodsList', data() { return { products: [ { id: 'GoodsDetail01', name: '多普勒效应马克杯', price: 35.50, image: require('../assets/1_DopplerEffect_多普勒效应_White.png') }, { id: 'GoodsDetail02', name: '透镜成像公式马克杯', price: 40.99, image: require('../assets/3_LensEquation_透镜成像公式_White.png') }, { id: 3, name: '黑洞温度马克杯', price: 32.00, image: require('../assets/5_BlackHoleTemperature_黑洞温度_White.png') }, { id: 4, name: '爱因斯坦场方程马克杯', price: 45.00, image: require('../assets/6_EinsteinFieldEquations_爱因斯坦场方程_White.png') } ], currentComponent: null } }, methods:{ // handleClick(){ // this.$emit('click', 'GoodsDetail01') //点击后跳转到 GoodsDetail01 页面 // } handleClick(id) { this.currentComponent = id } } } </script> <style> .product-wrap { width: 1200px; margin: 50px auto; display: flex; justify-content: space-between } .product { border: 1px solid #ececee; border-radius: 5px; cursor: pointer; transition: .5s; } .product:hover { border: 1px solid #979797; box-shadow: 0px 0px 7px #979797; } .product img { width: 270px; padding: 10px; border-bottom: 1px solid #ececee; } .product>div { display: flex; justify-content: space-between; align-items: center; padding: 0 10px; } </style>goodslist商品主页点击跳转到goodsdetail01和goodsdetail02,不能用路由,使用动态组件跳转写出全部代码
以下是使用动态组件实现商品主页点击跳转到详情页的完整代码:
```
<template>
<div class="product-wrap">
<div class="product" v-for="product in products" :key="product.id" @click="handleClick(product.id)">
<img :src="product.image" alt="">
<div>
<span>{{ product.name }}</span>
<h4>¥{{ product.price }}</h4>
</div>
</div>
<component :is="currentComponent"></component>
</div>
</template>
<script>
import GoodsDetail01 from './GoodsDetail01.vue'
import GoodsDetail02 from './GoodsDetail02.vue'
export default {
name: 'GoodsList',
components: {
GoodsDetail01,
GoodsDetail02
},
data() {
return {
products: [
{
id: 'GoodsDetail01',
name: '多普勒效应马克杯',
price: 35.50,
image: require('../assets/1_DopplerEffect_多普勒效应_White.png')
},
{
id: 'GoodsDetail02',
name: '透镜成像公式马克杯',
price: 40.99,
image: require('../assets/3_LensEquation_透镜成像公式_White.png')
},
{
id: 'GoodsDetail03',
name: '黑洞温度马克杯',
price: 32.00,
image: require('../assets/5_BlackHoleTemperature_黑洞温度_White.png')
},
{
id: 'GoodsDetail04',
name: '爱因斯坦场方程马克杯',
price: 45.00,
image: require('../assets/6_EinsteinFieldEquations_爱因斯坦场方程_White.png')
}
],
currentComponent: null
}
},
methods: {
handleClick(id) {
this.currentComponent = id
}
}
}
</script>
<style>
.product-wrap {
width: 1200px;
margin: 50px auto;
display: flex;
justify-content: space-between
}
.product {
border: 1px solid #ececee;
border-radius: 5px;
cursor: pointer;
transition: .5s;
}
.product:hover {
border: 1px solid #979797;
box-shadow: 0px 0px 7px #979797;
}
.product img {
width: 270px;
padding: 10px;
border-bottom: 1px solid #ececee;
}
.product > div {
display: flex;
justify-content: space-between;
align-items: center;
padding: 0 10px;
}
</style>
```
在该代码中,我们使用了动态组件来实现商品详情页的跳转。首先,在`<template>`标签内,我们通过`v-for`指令遍历`products`数组,动态生成商品卡片,并在卡片上绑定了`handleClick()`方法,该方法会在用户点击商品卡片时被调用,将`currentComponent`属性设置为当前商品的`id`,从而实现动态组件的切换。
在`<script>`标签内,我们首先通过`import`语句引入了两个商品详情页的组件。然后,在`components`属性中注册了这两个组件,以便在后面使用。接着,我们定义了`data`属性,其中包含了商品数组`products`和当前组件的名称`currentComponent`。`handleClick()`方法用于在用户点击商品卡片时设置`currentComponent`属性。
最后,在`<style>`标签内,我们定义了一些样式,用于美化商品卡片的外观。
阅读全文
相关推荐
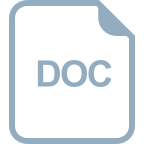
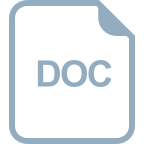
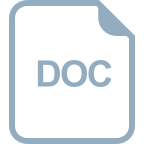
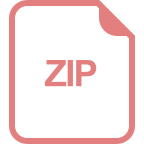
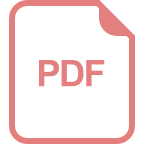
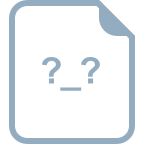
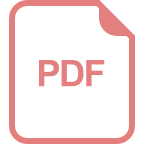
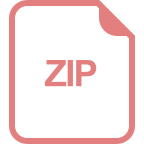
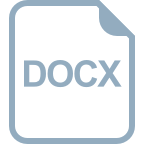
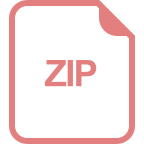
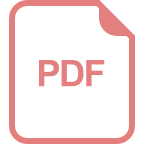
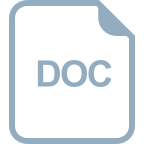
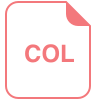
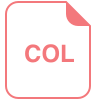
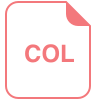
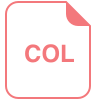
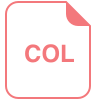