写一个cpp代码,生成一棵哈夫曼树,并进行编码和解码操作
时间: 2024-12-29 12:36:41 浏览: 22
在C++中,我们可以使用优先队列(`std::priority_queue`)和一个简单的节点结构来创建哈夫曼树。首先,我们定义节点类(HuffmanNode),然后实现编码、解码以及构建哈夫曼树的函数。这里是一个简化的示例:
```cpp
#include <queue>
#include <map>
// 定义节点类
struct HuffmanNode {
char data;
int freq;
HuffmanNode* left = nullptr;
HuffmanNode* right = nullptr;
HuffmanNode(char ch, int f) : data(ch), freq(f) {}
};
// 比较节点频率的比较函数
bool compare(HuffmanNode* a, HuffmanNode* b) {
return a->freq > b->freq;
}
// 构建哈夫曼树的函数
HuffmanNode* buildHuffmanTree(std::vector<HuffmanNode*>& nodes) {
if (nodes.empty()) return nullptr;
std::priority_queue<HuffmanNode*, std::vector<HuffmanNode*>, decltype(compare)> pq(compare);
for (HuffmanNode* node : nodes)
pq.push(node);
while (pq.size() > 1) {
HuffmanNode* left = pq.top(); pq.pop();
HuffmanNode* right = pq.top(); pq.pop();
HuffmanNode* newNode = new HuffmanNode('\0', left->freq + right->freq);
newNode->left = left;
newNode->right = right;
pq.push(newNode);
}
return pq.top(); // 返回根节点
}
// 编码和解码函数
std::string encode(const HuffmanNode* root, const char& input) {
std::string code;
HuffmanNode* current = root;
while (input != '\0') {
if (input == current->data) {
code += '1';
current = current->left;
} else {
code += '0';
current = current->right;
}
input--;
}
return code;
}
std::string decode(const HuffmanNode* root, const std::string& code) {
std::string result = "";
HuffmanNode* current = root;
for (char bit : code) {
if (bit == '1')
current = current->left;
else
current = current->right;
if (!current->left && !current->right) { // 如果遇到叶子节点,结束解码
result += current->data;
current = root; // 重新设置当前节点到根节点,准备下一个字符
}
}
return result;
}
int main() {
std::vector<HuffmanNode*> nodes = {/* 初始化一些有频率的字符节点 */ ...};
HuffmanNode* huffmanRoot = buildHuffmanTree(nodes);
// 示例编码和解码
std::string encodedCode = encode(huffmanRoot, 'a'); // 用字符'a'进行编码
std::string decodedString = decode(huffmanRoot, encodedCode); // 解码编码后的字符串
std::cout << "Encoded Code: " << encodedCode << std::endl;
std::cout << "Decoded String: " << decodedString << std::endl;
delete huffmanRoot; // 清理内存
return 0;
}
```
阅读全文
相关推荐




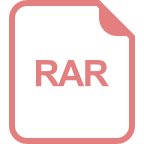

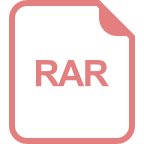








