springboot使用redis示例
时间: 2023-08-19 10:09:43 浏览: 108
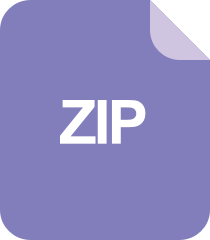
基于SpringBoot 操作redis Demo
下面是一个使用Spring Boot和Redis的示例:
首先,确保在你的Spring Boot项目中已经添加了Redis的依赖。你可以在 `pom.xml` 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
接下来,在你的Spring Boot应用程序中创建一个配置类,配置Redis连接信息。例如,在 `application.properties` 文件中添加以下配置:
```properties
spring.redis.host=localhost
spring.redis.port=6379
```
然后,你可以在任何需要使用Redis的地方注入`RedisTemplate`,并使用它进行操作。以下是一个简单的示例:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class RedisService {
@Autowired
private RedisTemplate<String, String> redisTemplate;
public void setValue(String key, String value) {
redisTemplate.opsForValue().set(key, value);
}
public String getValue(String key) {
return redisTemplate.opsForValue().get(key);
}
public void deleteValue(String key) {
redisTemplate.delete(key);
}
}
```
在上面的示例中,我们使用`RedisTemplate`进行了常见的操作,包括设置值、获取值和删除值。
最后,在你的控制器或其他地方使用`RedisService`来调用这些方法。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@Autowired
private RedisService redisService;
@GetMapping("/redis/set/{key}/{value}")
public String setValue(@PathVariable String key, @PathVariable String value) {
redisService.setValue(key, value);
return "Value set successfully";
}
@GetMapping("/redis/get/{key}")
public String getValue(@PathVariable String key) {
return redisService.getValue(key);
}
@GetMapping("/redis/delete/{key}")
public String deleteValue(@PathVariable String key) {
redisService.deleteValue(key);
return "Value deleted successfully";
}
}
```
通过上述示例,你可以通过访问 `/redis/set/{key}/{value}` 来设置值,通过访问 `/redis/get/{key}` 来获取值,通过访问 `/redis/delete/{key}` 来删除值。
这只是Redis在Spring Boot中的基本使用示例。你还可以使用其他功能,如哈希、列表、集合等。Spring Data Redis提供了更多的操作方法和注解,你可以根据自己的需求进行学习和使用。
阅读全文
相关推荐
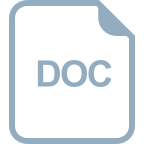
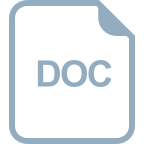
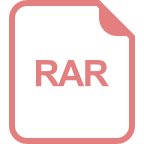
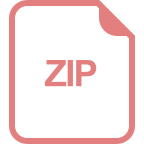
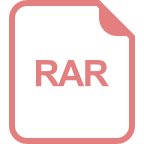
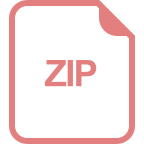
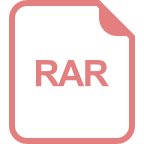
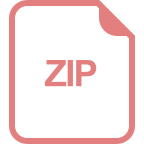
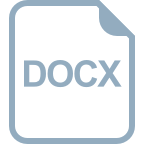
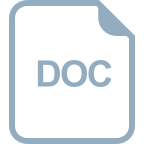







